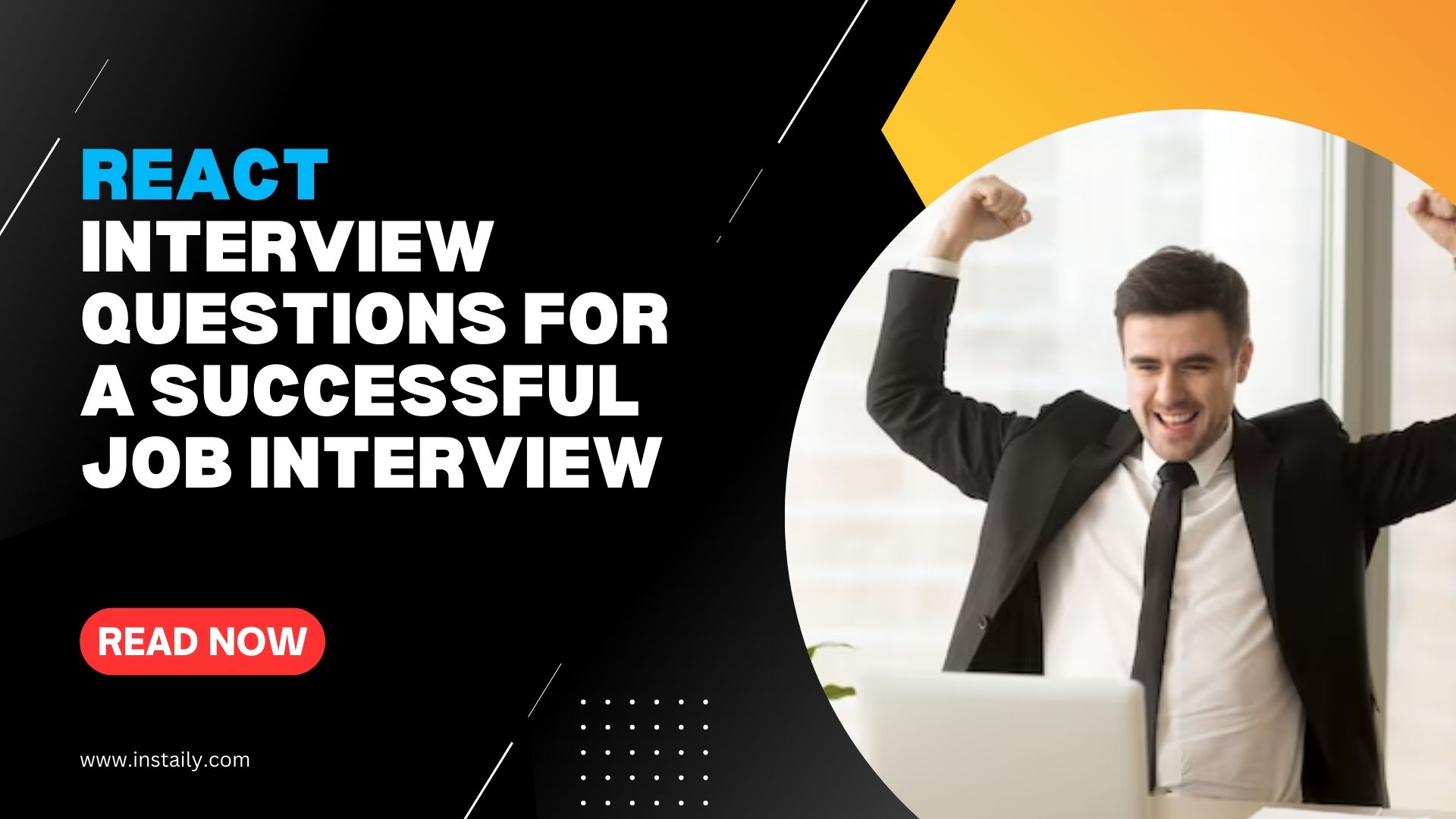
The Ultimate Guide to React Interview Questions and Answers for 2024
In this blog, We will share with you some of the most common and important React interview questions and answers that you may encounter in your next React job interview. React is a popular and powerful front-end JavaScript library that allows you to create interactive and reusable UI components for web and mobile applications. React has many features and advantages that make it a preferred choice for many developers and companies. However, React also has some challenges and limitations that you need to be aware of and overcome. Therefore, having a good knowledge of React concepts, principles, and best practices is essential for any React developer.
Your Road-map to Success: React Interview Questions and Answers
Here are some React interview questions and answers that we have prepared for you. We hope they will help you prepare well and ace your interview.
Q1. What is React?
Answer: React is a declarative, efficient, flexible open source front-end JavaScript library developed by Facebook in 2011. It follows the component-based approach for building reusable UI components, especially for single page applications. It is used for developing interactive view layer of web and mobile apps. It was created by Jordan Walke, a software engineer at Facebook. It was initially deployed on Facebook’s News Feed section in 2011 and later used in its products like WhatsApp & Instagram.
Q2. What are the advantages of using React?
Answer: Some of the advantages of using React are:
- React is easy to learn and use. It has a simple and expressive syntax that makes it easy to write and read code. It also has good documentation, tutorials, and community support that help developers learn and use React.
- React follows the MVC architecture. It is the V (view part) in the MVC (Model-View-Controller) architecture model and is referred to as “one of the JavaScript frameworks.” It is not fully featured but has many advantages of the open-source JavaScript User Interface (UI) library, which helps execute the task in a better manner.
- React uses Virtual DOM to improve efficiency. React uses virtual DOM to render the view. The virtual DOM is a virtual representation of the real DOM. Each time the data changes in a react app, a new virtual DOM gets created. Creating a virtual DOM is much faster than rendering the UI inside the browser. Therefore, with the use of virtual DOM, the efficiency of the app improves.
- Creating dynamic web applications is easy. In React, creating a dynamic web application is much easier. It requires less coding and gives more functionality. It uses JSX (JavaScript Extension), which is a particular syntax letting HTML quotes and HTML tag syntax to render particular subcomponents.
- React is SEO-friendly. React facilitates a developer to develop an engaging user interface that can be easily navigated in various search engines. It also allows server-side rendering, which is also helpful to boost the SEO of your app.
- React allows reusable components. React web applications are made up of multiple components where each component has its logic and controls.
Q3. What are the limitations of React?
Answer: Some of the limitations of React are:
- React only covers the view layer of the application, so you still need to choose other technologies to complete your stack.
- React requires a steep learning curve for beginners, especially if they are not familiar with ES6 syntax and concepts.
- React uses JSX, which is not a standard JavaScript syntax and may require a transpiler like Babel to convert it into plain JavaScript.
- React does not provide built-in support for animations, transitions, or styling. You need to use third-party libraries or tools to achieve these effects.
- React does not enforce any specific coding style or structure, so you need to follow best practices and conventions to maintain code quality and consistency.
Q4. What is useState() in React?
Answer: useState() is one of the built-in hooks in React that allows you to manage state in functional components. State is a set of data that determines how a component renders and behaves. useState() takes an initial state value as an argument and returns an array containing two elements: the current state value and a function to update it. You can use this function to change the state value by passing a new value or a function that returns a new value based on the previous state. For example:
import React, { useState } from 'react';
function Counter() {
// Declare a state variable called count and initialize it with 0
const [count, setCount] = useState(0);
// Define a function to increment the count by 1
function increment() {
setCount(count + 1);
}
// Define a function to decrement the count by 1
function decrement() {
setCount(count - 1);
}
// Return JSX code that renders a button with the count value
return (
<div>
<p>You clicked {count} times</p>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
);
}
Q5. What are keys in React?
Answer: Keys are special attributes that you can assign to elements in a list or an array to help React identify which items have changed, added, or removed. Keys should be unique and stable among the siblings in the same array. Keys help React optimize the rendering performance by minimizing the number of DOM operations. You can use any value as a key as long as it is unique and stable, such as an ID, a string, or a number. For example:
import React from 'react';
function List(props) {
// Get the items array from props
const items = props.items;
// Return JSX code that renders a list of items with keys
return (
<ul>
{items.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
Q6. What is JSX?
Answer: JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your React components. JSX is not a standard JavaScript syntax and requires a transpiler like Babel to convert it into plain JavaScript. JSX makes it easier to create and maintain UI components by combining the logic and the markup in one place. JSX also allows you to use JavaScript expressions inside curly braces to dynamically render data. For example:
import React from 'react';
function Greeting(props) {
// Get the name from props
const name = props.name;
// Return JSX code that renders a greeting message
return <h1>Hello, {name}!</h1>;
}
Q7. What are the differences between functional and class components?
Answer: Functional and class components are two ways of creating React components. Functional components are simple JavaScript functions that take props as an argument and return JSX code that renders the UI. Class components are ES6 classes that extend the React.Component class and have a render() method that returns JSX code that renders the UI. Class components also have access to other features such as state, lifecycle methods, and this keyword. For example:
import React from 'react';
// A functional component that takes props as an argument and returns JSX code
function Greeting(props) {
const name = props.name;
return <h1>Hello, {name}!</h1>;
}
// A class component that extends the React.Component class and has a render() method that returns JSX code
class Counter extends React.Component {
constructor(props) {
super(props);
// Initialize state with count value
this.state = {
count: 0,
};
// Bind the increment method to this
this.increment = this.increment.bind(this);
}
// Define a method to increment the count by 1
increment() {
this.setState((prevState) => ({
count: prevState.count + 1,
}));
}
// Define a lifecycle method to log the count value when the component updates
componentDidUpdate() {
console.log('The count is ' + this.state.count);
}
// Define a render() method that returns JSX code
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={this.increment}>+</button>
</div>
);
}
}
Some of the differences between functional and class components are:
- Functional components are simpler and easier to write and test than class components.
- Functional components do not have access to state, lifecycle methods, or this keyword by default, but they can use hooks to add these features.
- Class components have access to state, lifecycle methods, and this keyword by default, but they require more boilerplate code and binding methods.
- Functional components are more suitable for presentational or stateless components, while class components are more suitable for stateful or container components.
Q8. What is the virtual DOM? How does react use the virtual DOM to render the UI?
Answer: The virtual DOM is an in-memory representation of the real DOM that React uses to update the UI efficiently. The virtual DOM is a tree of objects that describe how the UI should look like. Each object represents an element or a component in the UI. The virtual DOM is faster than the real DOM because it does not involve any browser-specific operations or rendering.
React uses the virtual DOM to render the UI by following these steps:
- When a component’s state or props change, React creates a new virtual DOM tree that reflects the new UI.
- React compares the new virtual DOM tree with the previous one using a diffing algorithm and finds out which objects have changed.
- React updates only those objects that have changed in the real DOM using batched updates.
- The browser repaints and reflows the UI according to the changes in the real DOM.
Q9. What are the differences between controlled and uncontrolled components?
Answer: Controlled and uncontrolled components are two different ways to manage state in React components.
Controlled components
In a controlled component, the parent component manages the state of the component. The parent component passes down the current value of the state to the child component as a prop. The child component then uses this prop to render the UI. When the user interacts with the component, the parent component updates the state and passes the new value down to the child component.
Uncontrolled components
In an uncontrolled component, the component itself manages its own state. The component does this by listening for user events and updating its own state accordingly. The component then renders the UI based on its own state.
Here is a comparison between controlled and uncontrolled components:
Feature | Controlled component | Uncontrolled component |
State management | Parent component manages state | Component manages its own state |
Prop usage | Parent component passes down current value of state as prop | Component does not use props to manage state |
User interaction | Parent component updates state in response to user interaction | Component updates its own state in response to user interaction |
When to use controlled components:
- When you need to have complete control over the state of the component.
- When you need to validate user input.
- When you need to prevent the user from submitting invalid data.
When to use uncontrolled components:
- When you need the component to be more flexible and responsive to user interaction.
- When you need the component to be compatible with non-React code.
- When you need to use a third-party component that does not support controlled state.
Q10. What are props in React?
Answer: Props in React are immutable pieces of data that are passed from a parent component to its child components. Props are used to communicate data between components.
Props are immutable, which means that they cannot be changed by the child component. This is important because it ensures that the child component cannot accidentally modify the state of the parent component.
Props are typically used to pass data such as the title of a heading, the text of a button, or the value of a checkbox. Props can also be used to pass more complex data structures, such as an array of items or an object of configuration options.
To use props, the parent component simply passes the data to the child component as an argument. The child component can then access the props in its render() method.
Q11. Explain React state and props.
Answer: React state and props are two different ways to pass data between components in React.
State
State is mutable data that is owned by a component. It is used to represent the data that the component needs to render its UI. State can be updated by the component itself, or by its parent component.
To use state, the component must declare a state variable in its constructor. The state variable can then be updated using the setState() method.
Here is an example of a component that uses state to keep track of the number of times a button has been clicked:
class CounterComponent extends React.Component {
state = {
count: 0,
};
handleClick = () => {
this.setState({
count: this.state.count + 1,
});
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button
onClick={this.handleClick}>Click Me!</button>
</div>
);
}
}
In this example, the CounterComponent has a state variable called count. The count variable keeps track of the number of times the button has been clicked.
The CounterComponent also has a handleClick() method. This method is called when the button is clicked. The handleClick() method updates the count state variable and then re-renders the component.
Props
Props are immutable pieces of data that are passed from a parent component to its child components. Props are used to communicate data between components.
Props can be used to pass data such as the title of a heading, the text of a button, or the value of a checkbox. Props can also be used to pass more complex data structures, such as an array of items or an object of configuration options.
To use props, the parent component simply passes the data to the child component as an argument. The child component can then access the props in its render() method.
Here is an example of a parent component passing props to a child component:
const ParentComponent = () => {
return (
<ChildComponent title="My Title" />
);
};
const ChildComponent = ({ title }) => {
return <h1>{title}</h1>;
};
In this example, the ParentComponent passes the title prop to the ChildComponent. The ChildComponent then uses the title prop to render the heading.
When to use state and props:
- Use state when you need to manage data that is specific to the component.
- Use props to pass data from a parent component to a child component.
Here is a table summarizing the differences between state and props:
Feature | State | Props |
Ownership | Owned by the component | Owned by the parent component |
Mutability | Mutable | Immutable |
Usage | To manage data that is specific to the component | To pass data from a parent component to a child component |
Q12. Explain about types of side effects in React component.
Answer: There are two main types of side effects in React components:
- Imperative side effects
- Declarative side effects
Imperative side effects
- Imperative side effects are any operations that mutate state outside of the component, or that interact with the outside world, such as making an HTTP request or updating the DOM.
- Imperative side effects are typically triggered by a user event, such as clicking a button or submitting a form.
Declarative side effects
- Declarative side effects are side effects that are triggered by a change in state, such as updating the DOM or making an HTTP request.
- Declarative side effects are typically implemented using the
useEffect
hook.
Which type of side effect should you use?
In general, you should try to use declarative side effects whenever possible. Declarative side effects are easier to read and maintain, and they can help you to write more predictable code.
However, there are some cases where you may need to use imperative side effects. For example, you may need to use an imperative side effect to update the DOM directly, or to interact with a third-party library that does not support declarative side effects.
Feature | Imperative side effect | Declarative side effect |
Trigger | Typically triggered by a user event | Typically triggered by a change in state |
Implementation | Typically implemented using native JavaScript APIs | Typically implemented using the useEffect hook |
Pros | Can be more efficient | Easier to read and maintain |
Cons | Can be more difficult to read and maintain | Can be less efficient |
Q13. What is prop drilling in React?
Answer: Prop drilling is a common problem in React applications that occurs when data needs to be passed down through multiple levels of components. This can lead to code that is difficult to read and maintain.
Here is an example of prop drilling:
const ParentComponent = () => {
return (
<ChildComponent data={someData} />
);
};
const ChildComponent = ({ data }) => {
return (
<GrandChildComponent data={data} />
);
};
const GrandChildComponent = ({ data }) => {
// Use the data prop to render the UI
};
In this example, the data prop needs to be passed down through two levels of components before it can be used to render the UI. This is called prop drilling.
Prop drilling can make code difficult to read and maintain because it can be difficult to track where the data is coming from and how it is being used. It can also lead to code that is tightly coupled, which can make it difficult to test and refactor.
Q14. What are error boundaries?
Answer: Error boundaries are components that can catch errors and prevent them from crashing the entire application. They are useful for preventing errors from cascading through your application and causing other components to fail.
Error boundaries work by wrapping other components in the application. When an error occurs in one of the wrapped components, the error boundary catches the error and prevents it from propagating to the rest of the application.
Error boundaries can also be used to display a custom error message to the user and provide a way for the user to recover from the error.
To create an error boundary, you can use the ErrorBoundary
component provided by React. The ErrorBoundary
component takes a callback function as a prop. This callback function is called when an error occurs in one of the wrapped components.
Q15. What is React Hooks?
Answer: React Hooks are a new way to write React components. They allow you to use state and other React features without having to write a class component.
Hooks are functions that you can use to access and modify state, as well as perform other side effects, such as making HTTP requests or updating the DOM.
Hooks are a powerful tool that can help you to write more concise, reusable, and testable React components.
Here are some of the benefits of using React Hooks:
- Conciseness: Hooks allow you to write stateful logic in a more concise way than using class components.
- Reusability: Hooks are reusable, so you can use them to implement common functionality in your components.
- Testability: Hooks make components more testable, because you can isolate the stateful logic in your hooks and test them independently.
Q16. What are the rules that must be followed while using React Hooks?
Ans: The rules that must be followed while using React Hooks are:
- Only call Hooks at the top level. Don’t call Hooks inside loops, conditions, or nested functions. This ensures that Hooks are called in the same order each time a component renders, which is necessary for React to correctly preserve the state of Hooks between multiple useState and useEffect calls.
- Only call Hooks from React function components or custom Hooks. Don’t call Hooks from regular JavaScript functions or class components. This ensures that all stateful logic in a component is clearly visible from its source code and that Hooks have access to the React features they need.
These are the two main rules that must be followed while using React Hooks. If you violate these rules, you may encounter bugs or errors in your code.
Q17. What is the use of useEffect React Hooks?
Ans: The useEffect hook is a way to perform side effects in functional components, such as fetching data, updating the DOM, setting timers, etc. It runs after React has updated the UI and can be controlled by a dependency array. It can also return a cleanup function to run before the next side effect or when the component unmounts.
Q18. Why do React Hooks make use of refs?
Ans: React Hooks make use of refs for a few reasons:
- To access DOM elements. Refs allow you to access DOM elements directly from your functional components. This can be useful for things like focusing an input element or getting the current size of an element.
- To integrate with third-party libraries. Some third-party libraries require you to pass refs to their components. This is because they need to be able to access the DOM element in order to function properly.
- To manage imperative animations. Refs can be used to manage imperative animations in your functional components. This is because they allow you to access the DOM element that you need to animate.
Q19. What are Custom Hooks?
Ans: Custom Hooks are functions that you can create to encapsulate common functionality and share it between your components. They can be used to manage state, perform side effects, or access context data.
Custom Hooks are similar to built-in Hooks, but they are more powerful because they allow you to create your own custom functionality.
To create a Custom Hook, you simply need to create a function that starts with the word “use”. For example, you could create a custom Hook called useCounter
to manage the state of a counter in your components.
Q20. What are the differences between server-side rendering (SSR) and client-side rendering (CSR) in React?
Ans: Server-side rendering (SSR) and client-side rendering (CSR) are two different ways to render React applications.
Server-side rendering
In SSR, the React application is rendered on the server and the HTML output is sent to the client. This means that the user sees the fully rendered HTML page as soon as they load the application.
SSR has a number of advantages, including:
- Better performance: SSR can improve the performance of your application, especially for initial page loads. This is because the user does not have to wait for the JavaScript code to be downloaded and executed before they can see the page content.
- Better SEO: SSR is also better for SEO, as search engines can crawl and index the fully rendered HTML pages.
- Universal rendering: SSR allows you to render your application on both the server and the client, which can be useful for creating universal applications.
Client-side rendering
In CSR, the React application is rendered on the client. This means that the JavaScript code is downloaded and executed before the page is rendered.
CSR has a number of advantages, including:
- Simplicity: CSR is simpler to implement than SSR.
- Flexibility: CSR is more flexible than SSR, as you can use it to create dynamic and interactive applications.
- Bandwidth savings: CSR can save bandwidth, as the server only needs to send the JavaScript code to the client once.
Which one should you use?
The best way to choose between SSR and CSR is to consider the specific needs of your application. If you need an application with good initial page performance and SEO, then SSR is a good choice. If you need a more flexible and dynamic application, then CSR is a good choice.
Q21. How would you optimize a React application for performance?
Ans: To optimize a React application for performance, you can employ several strategies:
- Virtual DOM and Reconciliation: Utilize React’s virtual DOM to minimize direct manipulation of the actual DOM, allowing React to perform efficient updates by reconciling changes and then updating the DOM in batches.
- Component Optimization: Implement shouldComponentUpdate or React.memo to prevent unnecessary re-renders of components, particularly when there are no changes in the component’s props or state.
- Code Splitting and Lazy Loading: Break down your application into smaller chunks and load them only when needed using React.lazy and Suspense. This reduces the initial load time and improves the overall performance of the application.
- Memoization: Use memoization techniques for expensive function calls or computations to avoid redundant calculations and improve the application’s overall speed.
- Bundle Size Optimization: Minimize the bundle size by removing unused dependencies, optimizing imports, and utilizing tools like Webpack or Parcel to bundle and compress the code efficiently.
- Use of React Profiler and Performance Tools: Utilize React Profiler and other performance monitoring tools to identify performance bottlenecks, unnecessary re-renders, and components causing performance issues, and then optimize them accordingly.
- Proper State Management: Choose an appropriate state management solution like Redux or Context API based on the complexity of the application to efficiently manage the state and prevent unnecessary re-renders.
By implementing these strategies, you can significantly enhance the performance of your React application and ensure a smooth and efficient user experience.
Q22. How would you handle routing in a React application?
Ans: In a React application, you can handle routing using various techniques. One common approach is to use a dedicated routing library, such as React Router. Here’s how you can handle routing in a React application:
- Install React Router: Begin by installing React Router using npm or yarn:
npm install react-router-dom
- Set up Routes: Define different routes in your application using the
BrowserRouter
andRoute
components provided by React Router. For example:
import { BrowserRouter, Route, Switch } from 'react-router-dom';
function App() {
return (
<BrowserRouter>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} />
</Switch>
</BrowserRouter>
);
}
- Create Navigation Links: Build navigation links using the
Link
component from React Router. For instance:
import { Link } from 'react-router-dom';
// ...
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
- Handle Dynamic Routes: If your application requires dynamic routing, use parameters in your route definitions, such as:
<Route path="/users/:id" component={UserDetail} />
You can then access the parameter in the UserDetail
component using the useParams
hook provided by React Router.
- Nested Routes: To create nested routes, define child components within the parent component’s route definition. This allows you to manage nested UI components and their respective routes effectively.
By following these steps and utilizing the capabilities of React Router, you can easily handle routing in your React application, enabling smooth navigation between different views and components.
Q23. What is the purpose of the virtual DOM?
Ans: The virtual DOM (Document Object Model) is a lightweight copy of the actual DOM in memory that React uses for efficient rendering and updating of components. Its main purpose is to improve the performance of web applications by minimizing direct interaction with the actual browser DOM, which can be resource-intensive.
The key purposes of the virtual DOM are:
- Efficient Updates: When there are changes to the application state, React first updates the virtual DOM rather than the actual DOM. It then efficiently compares the updated virtual DOM with the previous version, identifying the necessary changes that need to be applied to the real DOM.
- Batched Updates: React can batch multiple updates to the virtual DOM and apply them in a single operation to the actual DOM, minimizing the number of actual DOM manipulations and thereby reducing the performance overhead.
- Faster Rendering: By using the virtual DOM, React can determine the most efficient way to update the actual DOM, ensuring that only the necessary components are re-rendered and updated, leading to faster and more efficient rendering of the UI.
- Cross-Platform Rendering: The virtual DOM enables React to efficiently handle complex UI components and easily render them on different platforms, making it an ideal choice for building cross-platform applications.
By leveraging the virtual DOM, React optimizes the process of updating the user interface, leading to improved performance, better user experience, and more efficient use of system resources.
Q24. What is the difference between a functional component and a class component?
Ans: The main differences between functional components and class components in React are as follows:
- Syntax: Functional components are written as JavaScript functions, while class components are created using JavaScript classes that extend the base
React.Component
class. - State Management: Class components have the ability to manage local state using the
this.state
mechanism, whereas functional components can manage state using theuseState
hook introduced in React 16.8 and later versions. - Lifecycle Methods: Class components support lifecycle methods such as
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
, which allow developers to perform actions during specific phases of a component’s lifecycle. Functional components, on the other hand, can utilize lifecycle methods by using theuseEffect
hook. - Code Complexity: Functional components tend to have simpler and more concise syntax, making them easier to read and maintain. Class components, due to their more verbose syntax and lifecycle methods, can often lead to more complex code.
- Performance: Functional components are generally more lightweight and performant than class components. This is because functional components can take advantage of React’s memoization and avoid unnecessary re-renders using the
React.memo
oruseMemo
hook. - Context and Refs: Class components can directly use the
this.context
property for consuming React context, while functional components can access context using theuseContext
hook. Similarly, class components handle refs using theReact.createRef()
method, whereas functional components use theuseRef
hook.
As React continues to evolve, functional components have become more powerful with the addition of hooks, which has made them the preferred choice for building components. However, class components are still prevalent in legacy codebases and may be necessary in certain situations, particularly when working with older versions of React or when dealing with complex component hierarchies.
Q25. What is the difference between a stateful and a stateless component?
Ans: The distinction between stateful and stateless components in React is as follows:
- Stateful Components: Also known as class components, stateful components manage their own state using the
this.state
mechanism. They can change their internal state usingsetState
and trigger re-renders when the state changes. Stateful components typically have more complex logic and can contain other components within them. - Stateless Components: Also referred to as functional components, stateless components do not have their own state and are primarily used for rendering UI based on the input props. They are simple functions that take props as an input and return React elements to render. They do not manage any state internally and do not possess lifecycle methods or the ability to manage local state.
Key differences between the two include:
- Complexity: Stateful components tend to be more complex due to the management of internal state and lifecycle methods, whereas stateless components are simple and focus solely on rendering based on the provided props.
- Performance: Stateless components are generally more lightweight and performant than stateful components, as they do not manage any state internally and do not trigger unnecessary re-renders. They can be optimized using techniques like memoization or React.memo to prevent re-render when props remain unchanged.
With the introduction of hooks in React, the distinction between stateful and stateless components has become less significant, as functional components can now manage state and use lifecycle methods through the use of hooks like useState, useEffect, useContext, and others. This has led to the preference for functional components in modern React development.
Q26. What is the difference between controlled and uncontrolled components?
Ans: The difference between controlled and uncontrolled components in React pertains to how they manage and handle data:
1. Controlled Components:
- Controlled components are those where form data is handled by a React component.
- The component controls and manages the state of the input elements within the form and updates the state when the user interacts with the input.
- The component explicitly sets the value of the input elements and provides an onChange event handler to update the state in response to user input.
2. Uncontrolled Components:
- Uncontrolled components are those where form data is handled by the DOM itself.
- The component does not manage the state of the input elements directly; instead, it allows the DOM to handle the form data.
- Data retrieval from uncontrolled components is typically done using references or DOM methods, such as
ref.current.value
.
Key differences between the two include:
- Data Handling: Controlled components manage form data through the component state, while uncontrolled components rely on the DOM to handle form data.
- Control Over Data: Controlled components offer greater control and flexibility over the form data, enabling validation and manipulation before updating the state. Uncontrolled components provide less direct control over the form data.
- Use Cases: Controlled components are often used when you need to perform actions such as validation, formatting, or to manage the form data before updating the state. Uncontrolled components are useful in cases where you want to allow the form to manage its own data without React’s direct involvement.
In general, controlled components are favored in React applications as they provide a more predictable and manageable way of handling form data, making it easier to implement complex form behaviors and validations.
Q27. What is prop drilling? How to avoid it?
Ans: Prop drilling, also known as “prop passing” or “component nesting“, is a common issue in React where you need to pass data from a top-level parent component down to a deeply nested child component through a series of intermediary components in the hierarchy. This can result in a less maintainable and clean codebase because many components have to receive and pass along props that they do not directly use, which can make the code harder to read and maintain.
To avoid prop drilling, you can use one or a combination of the following techniques:
- Context API: React’s Context API allows you to create a global state that can be accessed by any component within a component tree without having to explicitly pass props down through all intermediary components. This is especially useful for shared data, such as user authentication or theme settings.
- State Management Libraries: Use state management libraries like Redux or Mobx. These libraries provide a centralized store for application state that can be accessed by any component, eliminating the need to pass data through props.
- Higher-Order Components (HOCs): You can create higher-order components that wrap around components and pass down the required props. This can help avoid prop drilling by encapsulating the logic of passing props to child components.
- Render Props: Utilize the “render prop” pattern, where a component takes a function as a prop and calls that function with data that needs to be passed down. This allows you to avoid prop drilling by directly passing data to child components through the render prop.
- Hooks: If you’re using functional components, custom hooks can be an effective way to encapsulate state logic and avoid prop drilling. You can create custom hooks to manage and provide data to child components without the need to pass props explicitly.
- Component Composition: Instead of deeply nesting components, you can use component composition to build complex UIs by combining smaller, more focused components. This can help keep the component tree shallow and make prop drilling less of an issue.
Choose the technique that best fits your specific use case and the complexity of your application. In most cases, a combination of these approaches can help you avoid prop drilling and keep your codebase more maintainable and organized.
Q28. What is React Fiber? What benefits does it offer?
Ans: React Fiber is a new version of React’s core algorithm that was introduced in React 16. It is a complete rewrite of the previous reconciliation algorithm, which is the process of determining which parts of the UI need to be updated when the state changes. React Fiber aims to improve the performance and user experience of React applications, especially for complex and interactive UIs.
Some of the benefits of React Fiber are:
- Incremental rendering: React Fiber can split the rendering work into small chunks and spread it out over multiple frames, instead of blocking the main thread until the whole UI is updated. This allows React to pause, resume, or abort the rendering work as needed, and to prioritize the most important updates. This improves the responsiveness and smoothness of the UI, and avoids janky animations or input lag.
- Concurrency: React Fiber can handle multiple updates at the same time, and assign different priorities to them. For example, user interactions, such as clicks or keystrokes, have higher priority than background tasks, such as data fetching or code splitting. This allows React to render the UI in a consistent and predictable way, and to avoid unnecessary or outdated updates.
- New features: React Fiber enables new features and improvements that were not possible or easy to implement with the previous algorithm. For example, React Fiber supports fragments, portals, error boundaries, suspense, hooks, concurrent mode, and more.
Q29. What are Higher-Order Components (HOCs)? How do you use them?
Ans: Higher-order components (HOCs) are a technique in React for reusing component logic. They are not part of the React API, but a pattern that emerges from React’s compositional nature. A higher-order component is a function that takes a component as an argument and returns a new component that wraps the original component and adds some additional functionality or behavior.
HOCs can be used for various purposes, such as:
- Enhancing the functionality of a component, such as adding authentication, routing, or data fetching capabilities.
- Modifying the props or state of a component, such as adding or removing props, transforming props, or injecting state.
- Abstracting or manipulating the UI of a component, such as adding or removing elements, applying styles, or handling events.
To use HOCs in React, you typically follow these steps:
- Define the HOC function. This is a function that takes a component as input and returns a new component with additional functionality. For example:
const hoc = (WrappedComponent) => {
// ...
}
- Define the new component. This is a class or functional component that wraps the
WrappedComponent
and adds additional functionality. For example:
class NewComponent extends React.Component {
// ...
render() {
// ...
}
}
- Pass props to the
WrappedComponent
. In the render() method of theNewComponent
, pass all the props (including the additional props added by the HOC) to theWrappedComponent
. For example:
render() {
return <WrappedComponent {...this.props} additionalProp={additionalProp} />
}
- Return the new component. The HOC function should return the
NewComponent
so it can be used in the application. For example:
const hoc = (WrappedComponent) => {
class NewComponent extends React.Component {
// ...
render() {
// ...
}
}
return NewComponent;
}
- Use the HOC. You can use the HOC to wrap any component that you want to enhance or modify. For example:
const EnhancedComponent = hoc(OriginalComponent);
These are the basic steps to use HOCs in React.
Q30. What is Context API? How do you use it?
Ans: Context API is a feature of React that allows you to share data across multiple components without passing props manually. It is useful for passing data that is considered global or common for a group of components, such as theme, language, user, or authentication information.
To use Context API, you need to follow these steps:
- Create a context object using the React.createContext() method. This object contains two components: a Provider and a Consumer. The Provider component is used to provide the data to the components that need it, and the Consumer component is used to access the data from the Provider.
- Wrap the components that need the data in the Provider component, and pass the data as a value prop to the Provider. The Provider can be nested inside another Provider to override the data for a specific subtree of components.
- Use the Consumer component or the useContext() hook to read the data from the Provider. The Consumer component takes a function as a child that receives the data as an argument and returns a React element. The useContext() hook takes the context object as an argument and returns the data directly. You can use either of them depending on your preference and use case.
Conclusion,
This blog post has covered the most important React interview questions that you can expect to be asked in 2023. By understanding the key concepts of React and being able to answer these questions confidently, you will be well on your way to landing your dream React job. If you are serious about getting a React job, we encourage you to review the questions and answers in this blog post carefully. You should also practice answering these questions out loud so that you can deliver your answers confidently in an interview.