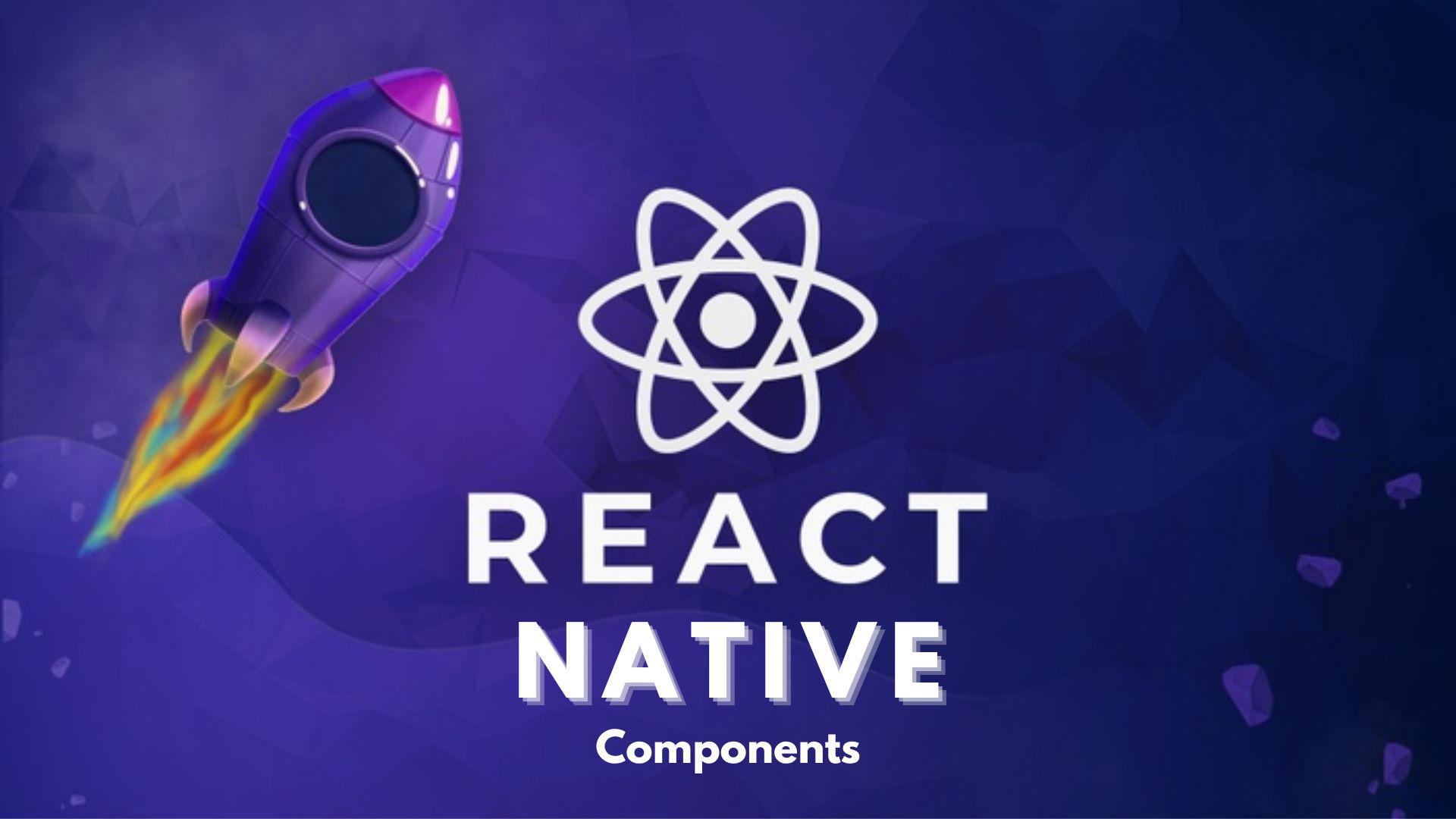
Mastering React Native Components, Props and State Concepts
React Native is a popular framework for building cross-platform mobile applications using JavaScript and React. It allows developers to create native-like user interfaces using reusable components, write code once and run it on both iOS and Android devices, and leverage the power and flexibility of React. In this blog post, we will cover some of the essential concepts that beginners need to know to get started with React Native, such as React Native components, props, and state.
What are React Native components?
Components are the building blocks of React Native applications. They are self-contained pieces of code that describe how a part of the user interface should look and behave. Components can be composed of other components, creating a hierarchy of components that form the application. Components can also have their own data and logic, which determine how they render and interact with the user.
There are two types of React Native components: class components and function components. Class components are defined using ES6 classes and have a render method that returns JSX, which is a syntax that looks like HTML but is actually JavaScript. Function components are defined using plain JavaScript functions and return JSX directly. Both types of components can accept props and use state, but they have some differences in how they handle lifecycle methods, hooks, and performance. You can learn more about the differences between class and function components in our React Native course.
Here is an example of a simple React Native component that displays a greeting message:
// A function component that takes a name prop and returns JSX
function Greeting(props) {
return (
<View>
<Text>Hello, {props.name}!</Text>
</View>
);
}
// A class component that takes a name prop and returns JSX
class Greeting extends React.Component {
render() {
return (
<View>
<Text>Hello, {this.props.name}!</Text>
</View>
);
}
}
What are props?
Props are short for properties. They are the way to pass data from one component to another. Props are read-only, which means that they cannot be modified by the component that receives them. Props are also static, which means that they do not change over time unless the parent component re-renders the child component with new props.
To pass props to a component, you can use attributes in JSX, similar to HTML attributes. For example, to pass a name prop to the Greeting component, you can write:
<Greeting name="Naruto" />
To access props in a component, you can use the props object, which contains all the props that the component receives. For example, to access the name prop in the Greeting component, you can write:
props.name
or
this.props.name
depending on whether you are using a function or a class component.
You can pass any type of data as props, such as strings, numbers, booleans, arrays, objects, functions, etc. You can also pass multiple props to a component, as long as they have different names. For example, to pass a name and an age prop to the Greeting component, you can write:
<Greeting name="Naruto" age={25} />
You can also use the spread operator (…) to pass props to a component, which can be useful when you have a lot of props or when you want to pass props from one component to another. For example, to pass all the props from the App component to the Greeting component, you can write:
function App(props) {
return <Greeting {...props} />;
}
What is state?
State is the way to manage dynamic data in a component. State is mutable, which means that it can be changed by the component that owns it. State is also local, which means that it is only accessible by the component that defines it and its children components.
To use state in a component, you need to initialize it and update it. There are two ways to do this: using the useState hook or using the setState method. The useState hook is a function that lets you create and update state in a function component. The setState method is a function that lets you create and update state in a class component.
The useState hook takes an initial state value as an argument and returns an array with two elements: the current state value and a function to update the state value. You can use array destructuring to assign names to these elements. For example, to create a state variable called count and a function to update it called setCount, you can write:
const [count, setCount] = useState(0);
The setState method takes an object or a function as an argument and merges it with the current state object. The object or the function should contain the state variables that you want to update and their new values. For example, to create a state object with a count variable and a function to update it, you can write:
this.state = {
count: 0,
};
this.setState({
count: this.state.count + 1,
});
or
this.setState((prevState) => ({
count: prevState.count + 1,
}));
To update the state value, you need to use the function that the useState hook or the setState method provides. You should never modify the state value directly, as this will not trigger a re-render and may cause errors. For example, to increment the count state value by one, you can write:
setCount(count + 1);
or
this.setState({
count: this.state.count + 1,
});
To access the state value, you can use the state variable or the state object, depending on whether you are using a function or a class component. For example, to access the count state value, you can write:
count
or
this.state.count
You can use the state value in your JSX, in your logic, or in your props. For example, to display the count state value in a Text component, you can write:
<Text>{count}</Text>
or
<Text>{this.state.count}</Text>
Here is an example of a simple component that uses state to implement a counter:
// A function component that uses the useState hook to create and update a count state value
function Counter() {
const [count, setCount] = useState(0);
return (
<View>
<Text>You clicked {count} times</Text>
<Button title="Click me" onPress={() => setCount(count + 1)} />
</View>
);
}
// A class component that uses the setState method to create and update a count state value
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
render() {
return (
<View>
<Text>You clicked {this.state.count} times</Text>
<Button
title="Click me"
onPress={() => this.setState({ count: this.state.count + 1 })}
/>
</View>
);
}
}
Conclusion
In this blog post, we have learned some of the essential concepts that beginners need to know to get started with React Native, such as components, props, and state. Components are the building blocks of React Native applications that describe how a part of the user interface should look and behave. Props are the way to pass data from one component to another. State is the way to manage dynamic data in a component. These concepts are fundamental to creating interactive and dynamic mobile applications with React Native.
1 Comment