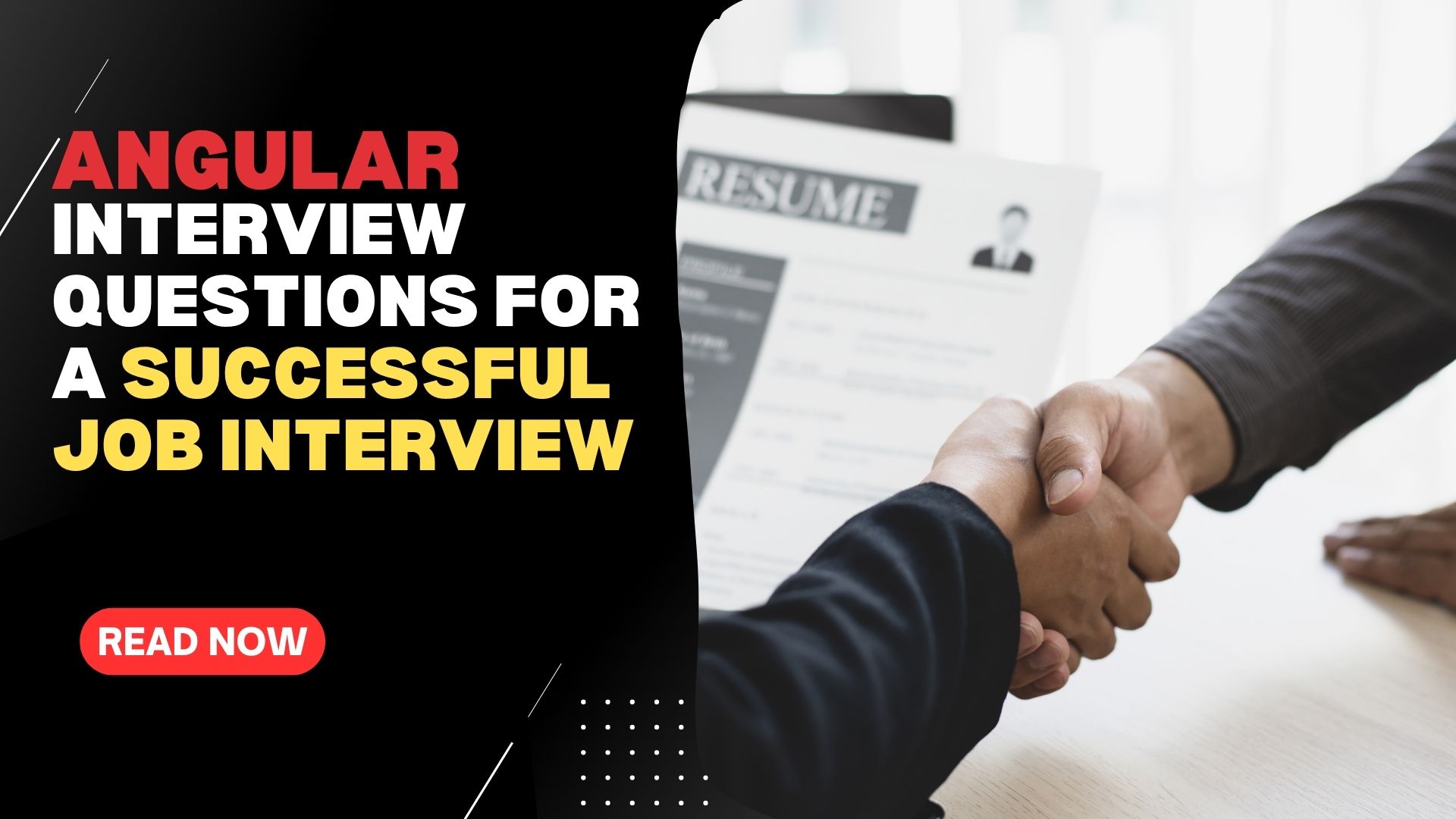
Top 30+ Angular Interview Questions and Answers for 2024
Imagine yourself sitting in an interview for your dream Angular role. The interviewer asks you questions, and you confidently answer it, demonstrating your deep understanding of the framework. You feel the interviewer being impressed, and you know that you’re one step closer to getting the job.
This is the scenario that you can create for yourself by preparing for your Angular interview in advance. And the best way to prepare is to familiarize yourself with the most common Angular interview questions and answers.
In this blog post, we will cover the top Angular interview questions and answers for 2024, along with tips on how to answer them effectively. We will also discuss some common mistakes that candidates make in Angular interviews, and how to avoid them.
Your Roadmap to Success: Angular Interview Questions and Answers
By reading this blog post and practicing your answers to the common interview questions, you can increase your chances of acing your Angular interview and landing your dream job.
Let’s dive in!
Q1. What is Angular?
Ans: Angular is an open-source web application framework developed by Google and a community of developers. It simplifies the creation of dynamic, single-page web applications (SPAs) by providing a structured and organized approach to front-end development. Angular uses TypeScript as its primary programming language and employs a component-based architecture, making it easier to build and maintain web applications. Key features include two-way data binding, dependency injection, and a powerful templating system. Angular also offers a rich ecosystem of libraries and tools, making it a popular choice for building modern web applications.
Learn how to build a progressive web app with Angular by taking our comprehensive course.
Q2. What are the benefits of using Angular?
Ans: Using Angular offers several benefits in web application development:
- Modular Development: Angular promotes a modular architecture, allowing developers to create self-contained components. This modularity enhances code reusability and maintainability.
- TypeScript Integration: Angular uses TypeScript, a statically typed superset of JavaScript. TypeScript helps catch errors during development, improving code quality and reducing runtime errors.
- Two-Way Data Binding: Angular’s two-way data binding simplifies the synchronization of data between the view and the model. This real-time updating of the user interface saves development time.
- Dependency Injection: Angular’s built-in dependency injection system makes it easy to manage dependencies and facilitates testing. It promotes the use of loosely coupled components, which enhances code maintainability.
- Powerful Templating: Angular provides a robust templating system that allows developers to create dynamic and interactive user interfaces with ease.
- Rich Ecosystem: Angular benefits from a vast ecosystem of libraries, tools, and extensions, making it suitable for various development needs.
- Cross-Platform Development: With tools like Angular Universal and NativeScript, Angular allows developers to create web and mobile applications using the same codebase.
- Active Community: Angular has a large and active community, resulting in extensive documentation, tutorials, and third-party support.
- Enhanced Performance: Angular has mechanisms like Ahead-of-Time (AOT) compilation and tree shaking, which help optimize the application’s performance.
- Angular CLI: The Angular Command Line Interface (CLI) streamlines project setup, code generation, and testing, improving development efficiency.
- Structured Code: Angular enforces best practices and a well-structured codebase, making it easier for development teams to collaborate and maintain projects.
Angular offers a robust and organized framework for web development, making it an excellent choice for building modern, scalable, and maintainable web applications.
Q3. What are the different components of an Angular application?
Ans: An Angular application is typically composed of several key components, each serving a specific purpose in the application’s structure and functionality. Here are the different components of an Angular application:
- Components: Components are the building blocks of an Angular application. They encapsulate the application’s logic and user interface. Each component is responsible for a specific part of the application and can contain its HTML template, CSS styles, and TypeScript code.
- Modules: Modules are used to organize an Angular application into cohesive units. Each module can include a set of related components, services, and other features. Angular applications usually have a root module, and developers can create additional modules to keep code organized.
- Services: Services are used to encapsulate business logic and share data or functionality across components. They are typically used for tasks like making HTTP requests, handling data, or performing other application-specific operations.
- Templates: Templates define the user interface (UI) of a component. They use Angular’s templating syntax to bind data from the component’s class to the view, allowing for dynamic rendering of content.
- Directives: Directives are special markers in the HTML that instruct Angular to modify or manipulate the DOM. Angular provides built-in directives like ngFor for rendering lists and ngIf for conditional rendering. Developers can also create custom directives for specific behaviors.
- Pipes: Pipes are used for data transformation and formatting in Angular templates. They allow developers to display data in a more user-friendly format. Angular provides built-in pipes like DatePipe and UpperCasePipe, and custom pipes can be created as needed.
- Routing: Routing is used to navigate between different views or components in a single-page application (SPA). Angular’s Router module provides tools for defining routes, handling navigation, and displaying the appropriate component based on the URL.
- Dependency Injection: Angular’s dependency injection system is a core feature that helps manage the dependencies of components and services. It allows for the injection of required services and other objects into components, making code more modular and testable.
- Metadata: Metadata is used to decorate classes and provide additional information to Angular. For example, the @Component decorator is used to specify metadata for components, including the selector, template, and styles.
- Interceptors: Interceptors are used to intercept HTTP requests and responses globally in an application. They provide a way to add custom logic, such as authentication or error handling, to HTTP requests.
These components work together to create a structured, modular, and maintainable Angular application. Developers can organize their code into reusable components, manage dependencies efficiently, and create dynamic user interfaces with ease.
Q4. What is data binding in Angular?
Ans: Data binding in Angular is a powerful feature that allows you to establish a connection between the user interface (UI) and the application’s data. It enables automatic synchronization of data between the two, ensuring that changes in one are reflected in the other without manual intervention. This two-way communication simplifies the development of dynamic and interactive web applications.
Angular supports four types of data binding:
1. Interpolation (One-Way Binding): Interpolation is a simple one-way data binding technique. It allows you to embed expressions within double curly braces {{ }} in the HTML template. These expressions are evaluated, and their values are displayed in the UI. Interpolation is mainly used to display data from the component’s class in the template.
Example:
<p>{{ message }}</p>
2. Property Binding (One-Way Binding): Property binding allows you to bind a property of an HTML element to a property of a component’s class. This one-way binding ensures that changes in the component class are reflected in the UI. Property binding is used for setting element properties like disabled, src, or innerHTML.
Example:
<button [disabled]="isDisabled">Click me</button>
3. Event Binding (One-Way Binding): Event binding allows you to bind an event in the HTML template to a method in the component’s class. When the event is triggered, the associated method is executed. Event binding is used for handling user interactions such as clicks, mouse events, or key presses.
Example:
<button (click)="onButtonClick()">Click me</button>
4. Two-Way Binding: Two-way binding combines property binding and event binding to achieve two-way communication between the UI and the component class. It’s typically used with form elements like <input> and <select>. Changes made in the UI are reflected in the component, and vice versa.
Example:
<input [(ngModel)]="userName">
Data binding simplifies the development process by reducing the need for manual DOM manipulation and ensures that the UI is always in sync with the application’s data. It’s a fundamental concept in Angular that enables the creation of dynamic and responsive user interfaces.
Q5. What are the different types of data binding in Angular?
Ans: In Angular, there are four main types of data binding:
Interpolation (One-Way Binding):
- Syntax: {{ expression }}
- Description: Interpolation is a one-way binding technique that allows you to display the result of an expression in the template. The expression is evaluated, and its value is inserted into the HTML at the location of the interpolation. It’s commonly used for displaying dynamic content in the view.
Example:
<p>{{ message }}</p>
Property Binding (One-Way Binding):
- Syntax: [property]=”expression”
- Description: Property binding allows you to set the value of an HTML element’s property to the value of an expression in the component. It’s a one-way binding from the component class to the template. Property binding is often used for manipulating element properties like disabled, src, or innerHTML.
Example:
<button [disabled]="isDisabled">Click me</button>
Event Binding (One-Way Binding):
- Syntax: (event)=”expression”
- Description: Event binding lets you listen for specific events (e.g., clicks, mouse events, keypresses) on an HTML element and trigger a method in the component when the event occurs. It’s a one-way binding from the template to the component and is used for handling user interactions.
Example:
<button (click)="onButtonClick()">Click me</button>
Two-Way Binding:
- Syntax: [(ngModel)]=”expression”
- Description: Two-way binding is a combination of property binding and event binding. It allows you to bind an input element’s value property to a component property and automatically updates both the component and the UI when either changes. Two-way binding is commonly used with form elements like <input> for bidirectional data flow.
Example:
<input [(ngModel)]="userName">
These data binding techniques are essential in Angular as they enable communication between the component class and the HTML template. They ensure that data is displayed and updated dynamically in response to user interactions or changes in the application’s state.
Q6. What is dependency injection in Angular?
Ans: Dependency injection (DI) is a fundamental concept in Angular that helps manage the dependencies of components and services within an application. It is a design pattern and a technique for achieving Inversion of Control (IoC) in software development.
In simpler terms, dependency injection is a way to provide the objects that a class (typically a component or a service) depends on, rather than the class creating those objects itself. This pattern promotes loose coupling between components and services, making the code more modular, maintainable, and testable.
Q7. What are the different types of dependency injection in Angular?
Ans: In Angular, there are three main types of dependency injection:
Constructor Injection:
- Description: Constructor injection is the most common type of dependency injection in Angular. It involves providing dependencies by declaring them as parameters in the constructor of a component or service class. Angular’s dependency injection system automatically creates instances of the required dependencies and passes them to the constructor when creating an instance of the class.
Example:
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-my-component',
template: '<p>{{ message }}</p>',
})
export class MyComponent {
message: string;
constructor(private dataService: DataService) { // Constructor injection
this.message = this.dataService.getData();
}
}
Setter Injection:
- Description: Setter injection involves providing dependencies through setter methods in a component or service class. In this approach, you define setter methods for the dependencies, and Angular’s injector injects the dependencies by calling these setter methods.
Example:
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-my-component',
template: '<p>{{ message }}</p>',
})
export class MyComponent {
message: string;
private dataService: DataService;
// Setter method for DataService injection
setDataService(dataService: DataService): void {
this.dataService = dataService;
this.message = this.dataService.getData();
}
}
Provider Injection:
- Description: Provider injection is less common and is typically used when you need to conditionally provide different implementations of a service. Instead of injecting the service directly into a component or service, you provide it using the providers array in a module or component’s metadata. This allows you to control the instance of the service for a specific part of your application.
Example:
import { Component, Injectable } from '@angular/core';
@Injectable()
export class DataService {
getData(): string {
return 'Data from DataService';
}
}
@Component({
selector: 'app-my-component',
template: '<p>{{ message }}</p>',
providers: [DataService], // Provider injection
})
export class MyComponent {
message: string;
constructor(private dataService: DataService) {
this.message = this.dataService.getData();
}
}
These three types of dependency injection in Angular provide flexibility in managing dependencies within your application. Constructor injection is the most commonly used approach, but setter injection and provider injection have their use cases when you need more control over how and when dependencies are provided.
Q8. What is routing in Angular?
Ans: Routing in Angular refers to the mechanism that allows you to navigate between different views or components within a single-page application (SPA). It enables users to move between different sections of your web application without the need for a full page reload. Angular’s built-in router provides a way to define and manage the application’s routes, handling navigation and displaying the appropriate component based on the URL.
Key concepts and features of routing in Angular include:
- Routes: Routes are defined as a collection of URL paths and their associated components. You configure these routes in your application to determine which component should be displayed when a specific URL is accessed.
- RouterOutlet: A router-outlet is a directive that acts as a placeholder where the routed component is displayed. When a route is activated, the component associated with that route is rendered within the router-outlet.
- Route Parameters: Routes can include parameters in the URL, allowing you to pass data from one view to another. For example, you can have a route like /product/:id where id is a parameter that represents the unique identifier of a product.
- RouterLink: The routerLink directive is used in templates to create navigation links. It generates the appropriate links based on the defined routes, making it easy to navigate between views within your application.
- Child Routes: Angular supports nested or child routes, allowing you to create complex routing structures. Child routes are defined within a parent route and can have their own components and templates.
- Guards: Route guards are used to control access to specific routes based on conditions. You can implement guards to ensure that a user is authenticated or has the necessary permissions to access certain views.
- Lazy Loading: Angular supports lazy loading of modules, which allows you to load modules and their associated components only when they are needed. This can significantly improve application performance by reducing initial loading times.
- Router Events: Angular’s router provides a set of events that you can subscribe to, allowing you to perform actions or handle specific scenarios during navigation, such as displaying loading indicators or tracking page views.
Routing in Angular is essential for building SPAs with multiple views and sections. It provides a seamless user experience by enabling navigation without the need for full page reloads. By defining routes, you can organize your application’s structure, control access to views, and create a logical flow for users as they interact with your web application.
Q9. What are the different types of routing in Angular?
Ans: In Angular, there are primarily two types of routing:
1. Traditional (Hash) Routing:
- URL Structure: Uses URLs with a hash (#) symbol followed by the route path. For example, http://example.com/#/products.
- Configuration: To use traditional routing, you configure the router with the RouterModule.forRoot() method and provide an array of route definitions.
- Advantages:
- Works well with older browsers that don’t support the HTML5 History API.
- Simpler server-side configuration because the hash fragment is not sent to the server.
- Disadvantages:
- URLs with hashes can be less user-friendly.
- SEO (Search Engine Optimization) can be more challenging because search engines may not index URLs with hashes.
- Slightly less clean URL structure.
2. HTML5 (Path) Routing:
- URL Structure: Uses clean URLs without hashes. For example, http://example.com/products.
- Configuration: HTML5 routing is configured similarly to traditional routing using the RouterModule.forRoot() method.
- Advantages:
- Cleaner and more user-friendly URLs.
- Better SEO as search engines can index clean URLs more effectively.
- Disadvantages:
- Requires server configuration to handle all possible route URLs, as the entire URL is sent to the server. This is often done using URL rewriting on the server.
In both traditional and HTML5 routing, the core routing concepts and features are the same. You define routes, create components for each route, use the <router-outlet> directive in your templates to display routed components, and utilize route parameters, guards, and other routing features as needed.
Q10. What are the different lifecycle hooks in Angular?
Ans: In Angular, there are several lifecycle hooks that allow you to tap into different stages of a component’s lifecycle. These hooks provide opportunities to perform actions or execute code at specific moments during the component’s creation, rendering, and destruction. Understanding these lifecycle hooks is crucial for managing component behavior and interactions effectively. Here are the main Angular lifecycle hooks:
ngOnChanges:
- Description: This hook is called whenever an input property of the component changes. It provides information about the previous and current values of the input properties.
- Usage: Useful for responding to changes in input properties and taking actions accordingly.
ngOnInit:
- Description: This hook is called once, immediately after the component and its input properties have been initialized. It’s commonly used for initialization logic, like fetching data from a service.
- Usage: Ideal for setting up the initial state of a component.
ngAfterViewInit:
- Description: This hook is called once, after the component’s view and child views (if any) have been initialized. It’s often used for DOM manipulation or working with child components.
- Usage: Useful for tasks that require the view to be fully rendered.
ngOnDestroy:
- Description: This hook is called just before a component is destroyed. It provides an opportunity to clean up resources, unsubscribe from observables, or perform other cleanup tasks.
- Usage: Important for preventing memory leaks and releasing resources when a component is no longer needed.
ngAfterContentInit:
- Description: This hook is called once, after Angular projects external content (like projected content from <ng-content>) into the component.
- Usage: Useful for working with content projected into a component and initializing content-related logic.
ngAfterContentChecked:
- Description: This hook is called after Angular checks the content projected into the component. It’s called whenever the content changes.
- Usage: Useful for performing checks or actions based on changes in projected content.
ngAfterViewChecked:
- Description: This hook is called after Angular checks the view and child views for changes. It’s called whenever the view changes.
- Usage: Useful for performing checks or actions based on changes in the component’s view.
ngDoCheck:
- Description: This hook is called during every change detection cycle, allowing you to implement custom change detection logic. It’s often used in conjunction with ngOnChanges.
- Usage: Useful for advanced scenarios where custom change detection is required.
These lifecycle hooks provide developers with fine-grained control over a component’s behavior at various stages of its lifecycle.
Q11. What is the difference between a component and a directive in Angular?
Ans: In Angular, components and directives are both building blocks for creating dynamic web applications, but they serve different purposes and have distinct characteristics.
components are used to create reusable UI elements with associated logic, while directives are used to add behavior or modify the appearance of HTML elements in templates. Components often have their templates, whereas directives work within existing templates.
Q12. What is the Angular CLI?
Ans: The Angular CLI, or Command Line Interface, is a powerful tool that simplifies the process of creating, developing, and deploying Angular applications. It provides a set of commands to automate various tasks, such as generating components, services, and modules, building and testing the application, and deploying it to production. It saves developers time and effort by setting up the project structure and configurations automatically.
Q13. What are the benefits of using the Angular CLI?
Ans: Using the Angular CLI offers several benefits:
- Project Setup: It quickly generates a well-structured Angular project with all the necessary files and configurations.
- Code Generation: It automates the creation of components, services, and modules, reducing repetitive tasks.
- Optimized Builds: The CLI optimizes the application’s build process, making it efficient for production.
- Testing: It simplifies running unit tests and end-to-end tests with built-in tools.
- Updates: It provides updates and best practices for Angular applications.
- Development Server: It offers a development server with live reloading for a seamless development experience.
Q14. What are some of the best practices for developing Angular applications?
Ans: Some best practices for Angular development include:
- Modularization: Organize the application into modules for better maintainability and separation of concerns.
- Reactive Programming: Embrace RxJS for handling asynchronous operations.
- Component-Based Architecture: Use components to encapsulate UI and logic.
- Services: Centralize data and business logic in services.
- Lazy Loading: Employ lazy loading for optimizing large applications.
- Change Detection: Be mindful of change detection strategies to optimize performance.
- Dependency Injection: Use Angular’s dependency injection for managing dependencies.
- TypeScript: Leverage TypeScript for strong typing and improved tooling.
- Code Linting: Apply code linting for consistent code style.
- Testing: Write unit tests and end-to-end tests to ensure code quality.
Q15. What is change detection in Angular?
Ans: Change detection is a core mechanism in Angular that tracks changes in the application’s state and updates the user interface accordingly. It ensures that the view reflects the most up-to-date data from the component’s model. Angular’s change detection system checks for changes in component properties and updates the DOM as needed.
Q16. How does change detection work in Angular?
Ans: Angular uses a tree of components to perform change detection. During each change detection cycle, Angular checks the component tree, starting from the root component, and looks for changes in properties or events. It then updates the DOM to reflect these changes. This process continues until no more changes are detected.
Q17. What are the different types of change detection in Angular?
Ans: Angular supports two types of change detection:
- Default (Zone.js): In this mode, Angular automatically triggers change detection after most asynchronous operations, such as HTTP requests, mouse events, or timers. This ensures that the UI remains in sync with the application’s state.
- OnPush: This mode allows developers to manually trigger change detection by using the ChangeDetectorRef when specific conditions are met. It’s useful for optimizing performance by reducing unnecessary change detection cycles.
Q18. What is the Angular compiler?
Ans: The Angular compiler is a key part of the Angular framework responsible for translating Angular components, templates, and metadata into JavaScript code that can be executed by the browser. It performs ahead-of-time (AOT) compilation, which compiles templates and components during the build process, resulting in smaller bundle sizes and improved runtime performance. AOT compilation also helps catch template errors during development, reducing runtime errors in production.
Q19. How does the Angular compiler work?
Ans: The Angular compiler is a two-step process:
- Ahead-of-Time (AOT) Compilation: During the build process, Angular’s AOT compiler translates templates and components into optimized JavaScript code, eliminating the need for template compilation in the browser. This reduces bundle sizes and improves runtime performance.
- Just-in-Time (JIT) Compilation (Development Only): In development mode, Angular uses JIT compilation, where templates are compiled in the browser. While less efficient than AOT, JIT compilation provides a faster development turnaround.
Q20. What are the different types of Angular templates?
Ans: Angular supports three types of templates:
- Inline Templates: Templates defined directly within the component’s metadata using the template property.
- External Templates: Templates stored in separate HTML files and referenced in the component’s metadata using the templateUrl property.
- Dynamic Templates: Templates generated at runtime using the ComponentFactoryResolver. These are useful for dynamic component creation.
Q21. What are the different types of Angular directives?
Ans: Angular directives are categorized into two main types:
- Attribute Directives: These change the appearance or behavior of DOM elements by manipulating their attributes. Examples include ngClass, ngStyle, and custom attribute directives.
- Structural Directives: These modify the structure of the DOM by adding or removing elements. Examples include ngIf, ngFor, and custom structural directives.
Q22. What are the different types of Angular pipes?
Ans: Angular provides several built-in pipes for transforming data in templates:
- Pure Pipes: These are stateless and return a new value when the input data changes. Examples include date, uppercase, and custom pure pipes.
- Impure Pipes: These can have side effects and are re-evaluated with every change detection cycle. Use them with caution. Angular doesn’t provide impure pipes by default, but you can create custom ones.
Q23. What are the different types of Angular services?
Ans: Angular services can be categorized based on their purpose:
- Data Services: Handle data retrieval and storage, such as HTTP services for API calls or local data storage services.
- Business Services: Implement application-specific logic and facilitate communication between components.
- Utility Services: Provide utility functions, such as authentication services or logging services.
- Shared Services: Store and share state or data between multiple components or modules.
- Feature Services: Serve a specific feature or functionality within an application.
Q24. What is Angular Material?
Ans: Angular Material is a UI component library for Angular applications that follows Google’s Material Design guidelines. It provides a set of pre-designed, customizable UI components, including buttons, inputs, navigation menus, and more. Angular Material simplifies the process of creating visually appealing and consistent user interfaces in Angular applications.
Q25. What are the benefits of using Angular Material?
Ans: Using Angular Material offers several advantages:
- Consistent Design: It ensures a consistent, visually appealing design following Material Design principles.
- Pre-designed Components: Angular Material provides a wide range of UI components that are easy to use and customize.
- Accessibility: Components are designed with accessibility in mind, making applications more inclusive.
- Responsive: Components are built to be responsive and adapt to various screen sizes.
- Theming: It allows for easy theming and customization to match the application’s brand.
- Community Support: Angular Material has a large community and is actively maintained, ensuring long-term support and updates.
Q26. What are some of the best practices for using Angular Material?
Ans: Best practices for using Angular Material include:
- Theming: Customize themes to match the application’s branding.
- Lazy Loading: Use lazy loading for Angular Material modules to optimize bundle sizes.
- Material Modules: Organize Angular Material imports into dedicated modules.
- Accessibility: Ensure components are used in an accessible way and test with assistive technologies.
- Responsive Design: Utilize Angular’s responsive layout features alongside Angular Material components for responsiveness.
Q27. What is the Angular Forms module?
Ans: The Angular Forms module provides tools and services for building and working with forms in Angular applications. It includes form controls, validators, and form groups to create complex forms with data binding, validation, and user interaction handling.
Q28. What are the different types of Angular forms?
Ans: Angular supports two types of forms:
- Template-driven Forms: These are built using template-driven directives, primarily using ngModel for two-way data binding. They are suitable for simple forms.
- Reactive Forms: These are built programmatically using the FormControl, FormGroup, and FormArray classes. Reactive forms offer more flexibility and are suitable for complex forms and dynamic scenarios.
Q29. What are some of the best practices for using Angular forms?
Ans: Best practices for using Angular forms include:
- Reactive Forms: Use reactive forms for complex and dynamic forms.
- Validation: Implement both template-driven and reactive form validation to ensure data integrity.
- FormGroup: Organize form controls into FormGroup and FormArray structures for better organization.
- FormBuilder: Use the FormBuilder service to simplify the creation of form controls and groups.
- Submit Handling: Handle form submissions with RxJS observables for better control and asynchronous operations.
- Unit Testing: Write unit tests for forms to ensure they function correctly.
- Custom Validators: Create custom validators for specific form validation rules.
- Error Handling: Handle form errors and provide meaningful error messages to users.
Q30. What is Angular Universal?
Ans: Angular Universal is a technology and framework for server-side rendering (SSR) in Angular applications. SSR is a technique that allows the initial rendering of a web page to occur on the server rather than in the browser. Angular Universal extends the capabilities of Angular to enable server-side rendering, offering several advantages:
- Improved SEO: By rendering the initial HTML on the server, Angular Universal improves search engine optimization (SEO). Search engine bots can crawl and index the content more effectively since the HTML is readily available.
- Faster Initial Page Load: SSR reduces the time it takes for the initial page to load because the server sends pre-rendered HTML to the browser. Users see content sooner, resulting in a better user experience, particularly on slower network connections.
- Optimized Social Sharing: When sharing links on social media platforms, having pre-rendered HTML makes the content appear correctly in social previews, including titles, descriptions, and images.
- Accessibility: Pre-rendered content is immediately accessible to users with disabilities or assistive technologies, improving accessibility compliance.
- Improved Performance: SSR can reduce the load on the client’s device, especially on low-powered devices, by offloading some rendering work to the server.
- Dynamic Data: Angular Universal can still render pages with dynamic data. It can fetch data from APIs on the server and include it in the pre-rendered HTML.
Angular Universal works by running Angular on the server (Node.js) to generate the initial HTML response for a requested URL. Once the initial rendering is complete, the application continues to run on the client, and further interactions occur in the browser using the Angular framework.
Q31. What is TypeScript?
Ans: TypeScript is a statically typed superset of JavaScript that adds optional static typing and several other features to the language. Developed and maintained by Microsoft, TypeScript is designed to make JavaScript development more robust, maintainable, and scalable, especially for larger and complex applications.
Q32. What are Single Page Applications (SPA)?
Ans: Single Page Applications (SPAs) are a type of web application that operates within a single web page. Unlike traditional multi-page applications (MPAs), where each interaction with the web application involves loading an entirely new page from the server, SPAs load only once, and subsequent interactions dynamically update the content on that single page. This approach provides a more fluid and seamless user experience.
Q33. Differentiate between Angular and AngularJS
Ans: Angular and AngularJS are two different versions of the same front-end web development framework. Angular was released in 2016 as a complete rewrite of AngularJS, and it includes a number of significant changes.
Here is a table that summarizes the key differences between Angular and AngularJS:
Feature | Angular | AngularJS |
Programming language | TypeScript | JavaScript |
Component-based | Yes | No |
Routing | Built-in | Third-party libraries |
Dependency injection | Hierarchical | Flat |
Testing | Built-in | Third-party libraries |
Performance | Faster | Slower |
Community support | Active | Less active |
Q34. What are decorators in Angular?
Ans: Decorators in Angular are functions that can be used to modify the behavior of classes, properties, and methods. They are a powerful tool that can be used to extend the functionality of Angular and to write more concise and idiomatic code.
Decorators are defined using the ‘@’ symbol, followed by the name of the decorator function. For example, the following code defines a decorator function called MyDecorator:
function MyDecorator(target: any, propertyKey: string | symbol) {
// Do something with the target class, property, or method.
}
Conclusion,
In this blog, we have covered a wide range of Angular interview questions, from basic to advanced level. We hope you have found this information helpful and informative.
If you are preparing for an Angular interview, we recommend that you review these questions and make sure that you have a good understanding of the concepts covered. You may also want to practice answering the questions aloud to help you prepare for the real thing.
We wish you all the best in your Angular interview!
Tag:angular advanced interview questions, angular basic interview questions, angular forms interview questions, angular interview question, angular interview questions, angular interview questions 2023, angular interview questions and answers, angular material interview questions, angular questions, common angular interview questions, reactive forms in angular interview questions, top angular interview questions and answers