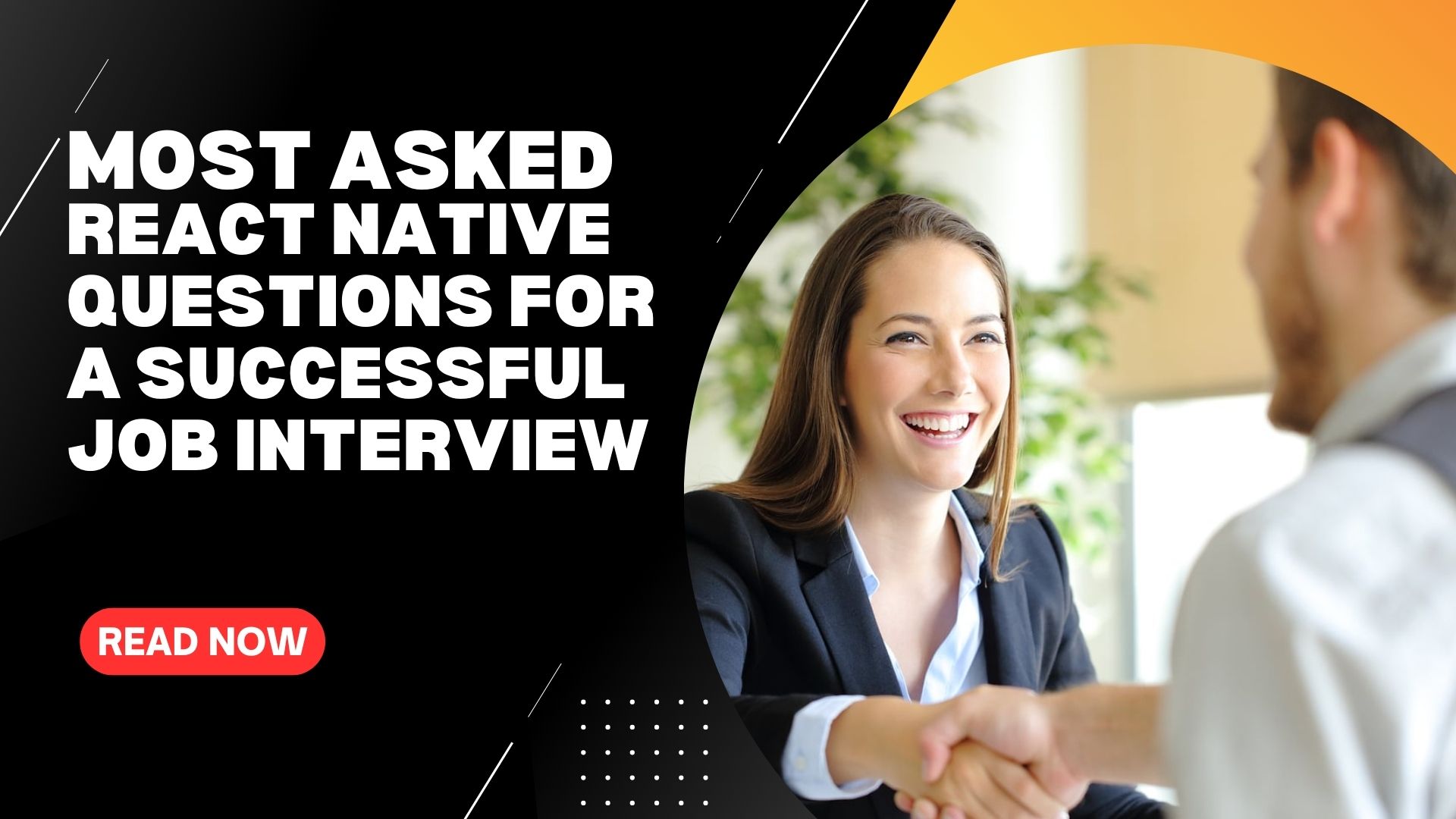
Top 40 React Native Interview Questions & Answers for 2024
Are you ready to embark on a journey that could potentially transform your career in app development? Are you intrigued by the world of React Native and eager to showcase your expertise in job interviews? If your answer is yes, you’ve landed on the right page.
In today’s tech-driven world, mobile app development is a rapidly growing field, and React Native has emerged as a star player. Whether you’re a seasoned developer looking to upskill or a fresh-faced coder just starting, mastering React Native is a smart move. But how do you ensure you’re well-prepared for those crucial job interviews? The answer lies in our ultimate guide: React Native Interview Questions in 2023.
The Journey Begins
Before we dive into the exciting world of React Native interview questions, let’s take a moment to understand why this framework is creating such a buzz.
React Native is an open-source framework developed by Facebook, designed to simplify the process of building mobile applications for both iOS and Android. What makes it unique is its use of JavaScript and React to create truly native experiences. With React Native, you can write code once and use it for multiple platforms, saving time and resources.
(That’s the reason why most of the job seekers who want to make a career in web development joined our React Native Course.)
But why is it important for you to prepare for React Native interviews? The answer is simple: in the competitive world of app development, demonstrating your expertise in React Native can set you apart from the crowd.
Your Roadmap to Success: React Native Interview Questions and Answers
Get ready to embark on a journey of learning and self-improvement. Below, we’ve categorized React Native interview questions into various sections to ensure you have a well-rounded understanding of this framework.
1. What is React Native?
Answer: React Native is a framework for building native mobile applications using JavaScript and React. It allows developers to use the same codebase for iOS and Android platforms, and leverage the power of React to create declarative and reusable UI components.
2. What are the benefits of using React Native?
Answer: Some of the benefits of using React Native are:
- Cross-platform compatibility: React Native enables developers to write one codebase that can run on both iOS and Android devices, saving time and resources.
- Hot reloading and live reloading: React Native supports hot reloading and live reloading, which means that developers can see the changes in their code reflected on the device screen without rebuilding the app or losing the app state.
- Native performance and look and feel: React Native uses native UI components and modules that interact with the native platform, resulting in high performance and native-like user experience.
- Large and active community: React Native has a large and active community of developers, contributors, and supporters, who provide helpful resources, libraries, tools, and feedback.
- Easy integration with existing code: React Native can be easily integrated with existing native code, allowing developers to use the best of both worlds.
3. What are the limitations of using React Native?
Answer: Some of the limitations of using React Native are:
- Lack of native features: React Native does not support all the native features and functionalities that are available on iOS and Android platforms, such as advanced animations, gestures, camera, etc. Developers may need to use third-party libraries or write native modules to access these features.
- Debugging challenges: Debugging React Native apps can be challenging, especially when dealing with native errors or performance issues. Developers may need to use different tools and techniques to debug different parts of the app.
- Compatibility issues: React Native may not be compatible with some older versions of iOS and Android devices, or some third-party libraries or services. Developers may need to test their apps extensively on different devices and platforms to ensure compatibility.
- Learning curve: React Native requires developers to have a good knowledge of JavaScript, React, and native development. Developers may need to learn new concepts, tools, and best practices to use React Native effectively.
4. What are the differences between React and React Native?
Answer: React and React Native are both frameworks for building user interfaces using JavaScript and React, but they have some key differences:
- Platform: React is a web framework that runs on browsers, while React Native is a mobile framework that runs on iOS and Android devices.
- UI components: React uses HTML elements and CSS styles to create UI components, while React Native uses native UI components that are rendered by the native platform.
- DOM manipulation: React uses a virtual DOM to manipulate the real DOM in the browser, while React Native does not use a DOM at all, but communicates with the native platform through a bridge.
- Styling: React uses CSS or CSS-in-JS libraries to style UI components, while React Native uses a subset of CSS properties and a flexbox layout system to style UI components.
5. What is Virtual DOM?
Answer: Virtual DOM is a concept that is used by React to improve the performance and efficiency of rendering UI components. Virtual DOM is an in-memory representation of the real DOM that is created by React. Whenever there is a change in the state or props of a component, React compares the new virtual DOM with the old virtual DOM, and calculates the minimum number of changes required to update the real DOM. This process is called reconciliation. Virtual DOM helps to avoid unnecessary re-rendering of UI components, which can improve the speed and user experience of web applications.
6. How does React Native work?
Answer: React Native works by using JavaScript and React to create UI components that are rendered by the native platform. React Native uses a JavaScript engine (such as JavaScriptCore or Hermes) to run the JavaScript code on a separate thread from the main UI thread. React Native also uses a bridge (such as MessageQueue or TurboModules) to communicate between the JavaScript thread and the native thread, and to access native modules and components. React Native leverages the power of React to create declarative and reusable UI components, and to manage the state and lifecycle of the components.
7. What are the core components in React Native?
Answer: The core components in React Native are the basic UI elements that are provided by the framework, such as View, Text, Image, Button, ScrollView, FlatList, etc. These core components are cross-platform, which means that they can run on both iOS and Android devices with the same code. The core components can also be styled and composed to create custom UI components.
8. What are the native components in React Native?
Answer: The native components in React Native are the UI elements that are specific to a certain platform, such as DatePickerIOS, SwitchAndroid, StatusBarIOS, etc. These native components can only run on their respective platforms, and they may have different props and behaviors than the core components. The native components can be used to access some native features and functionalities that are not available in the core components.
9. What is JSX?
Answer: JSX is a syntax extension for JavaScript that allows developers to write HTML-like code in their JavaScript files. JSX is used by React and React Native to create UI components in a declarative and expressive way. JSX is not a valid JavaScript code, but it is transformed into JavaScript code by a compiler (such as Babel) before execution.
10. How to style a React Native app?
Answer: There are different ways to style a React Native app, but the most common way is to use the StyleSheet API that is provided by the framework. The StyleSheet API allows developers to create style objects that contain CSS-like properties and values, such as color, fontSize, margin, padding, etc. The style objects can be applied to UI components using the style prop. The StyleSheet API also supports some features such as inheritance, media queries, pseudo-selectors, etc.
11. How to create a React Native app?
Answer:There are different ways to create a React Native app, but the most common way is to use the React Native CLI (Command Line Interface) that is provided by the framework. The React Native CLI allows developers to create a new React Native project with a simple command:
npx react-native init <projectName>
This command will generate a basic React Native app structure with some boilerplate code and dependencies. The React Native CLI also supports some options and templates to customize the app creation process.
12. How to handle state in React Native?
Answer: State is a term that refers to the data or information that is used by a UI component to render its content and behavior. State can be changed by user interactions, network requests, timers, etc. Handling state in React Native is similar to handling state in React, as both frameworks use the same concepts and principles. There are different ways to handle state in React Native, but the most common way is to use the useState hook that is provided by the framework.
The useState hook allows developers to create and update state variables in a functional component, and re-render the component when the state changes. The useState hook takes an initial value as an argument, and returns an array with two elements: the current state value and a function to update the state value. For example:
import React, { useState } from 'react';
import { View, Text, Button } from 'react-native';
const Counter = () => {
// Create a state variable called count with an initial value of 0
const [count, setCount] = useState(0);
// Define a function to increment the count by 1
const increment = () => {
setCount(count + 1);
};
// Define a function to decrement the count by 1
const decrement = () => {
setCount(count - 1);
};
// Return a UI component that displays the count and two buttons
return (
<View>
<Text>Count: {count}</Text>
<Button title="+" onPress={increment} />
<Button title="-" onPress={decrement} />
</View>
);
};
export default App;
13. How to use Redux in React Native?
Answer: Redux is a popular state management library that can be used in React Native apps to manage complex and shared state across multiple components. Redux works by using three main concepts: actions, reducers, and store. Actions are plain JavaScript objects that describe what happened in the app, such as user interactions, network requests, etc. Reducers are pure functions that take the previous state and an action as arguments, and return the next state based on the action type and payload.
Store is an object that holds the whole state of the app, and allows components to access and update the state using dispatch and subscribe methods. To use Redux in React Native, developers need to install redux and react-redux packages, and follow these steps:
• Define the initial state of the app as an object.
• Define the action types as constants or strings.
• Define the action creators as functions that return actions.
• Define the reducer as a function that takes the state and action as arguments, and returns the next state using a switch statement or an object lookup.
• Create the store using the createStore function from redux, and pass the reducer as an argument.
• Wrap the root component of the app with the Provider component from react-redux, and pass the store as a prop.
• Connect the components that need to access or update the state with the connect function from react-redux, and pass mapStateToProps and mapDispatchToProps functions as arguments.
• Use useSelector and useDispatch hooks from react-redux to access or update the state in functional components.
For example:
// Import redux and react-redux packages
import { createStore } from 'redux';
import { Provider, connect, useSelector, useDispatch } from 'react-redux';
// Define initial state
const initialState = {
counter: 0,
};
// Define action types
const INCREMENT = 'INCREMENT';
const DECREMENT = 'DECREMENT';
// Define action creators
const increment = () => {
return {
type: INCREMENT,
};
};
const decrement = () => {
return {
type: DECREMENT,
};
};
// Define reducer
const reducer = (state = initialState, action) => {
switch (action.type) {
case INCREMENT:
return {
...state,
counter: state.counter + 1,
};
case DECREMENT:
return {
...state,
counter: state.counter - 1,
};
default:
return state;
}
};
// Create store
const store = createStore(reducer);
// Define class component that uses connect function
class CounterClass extends React.Component {
render() {
// Destructure props
const { counter, increment, decrement } = this.props;
// Return UI component
return (
<View>
<Text>Count: {counter}</Text>
<Button title="+" onPress={increment} />
<Button title="-" onPress={decrement} />
</View>
);
}
}
// Define mapStateToProps function
const mapStateToProps = (state) => {
return {
counter: state.counter,
};
};
// Define mapDispatchToProps function
const mapDispatchToProps = (dispatch) => {
return {
increment: () => dispatch(increment()),
decrement: () => dispatch(decrement()),
};
};
// Connect class component with store
const CounterClassContainer = connect(
mapStateToProps,
mapDispatchToProps
)(CounterClass);
// Define functional component that uses hooks
const CounterFunction = () => {
// Use useSelector hook to access state
const counter = useSelector((state) => state.counter);
// Use useDispatch hook to dispatch actions
const dispatch = useDispatch();
// Return UI component
return (
<View>
<Text>Count: {counter}</Text>
<Button title="+" onPress={() => dispatch(increment())} />
<Button title="-" onPress={() => dispatch(decrement())} />
</View>
);
};
// Define root component that uses Provider component
const App = () => {
return (
<Provider store={store}>
<View>
<CounterClassContainer />
<CounterFunction />
</View>
</Provider>
);
};
export default App;
14. How to test a React Native app?
Answer: Testing is an important part of developing a React Native app, as it helps to ensure the quality, functionality, and performance of the app. Testing a React Native app can be done at different levels, such as unit testing, integration testing, end-to-end testing, etc. There are different tools and frameworks that can be used to test a React Native app, such as Jest, Enzyme, React Native Testing Library, Detox, Appium, etc. These tools and frameworks have different features and capabilities, such as mocking, snapshot testing, code coverage, automation, etc.
To test a React Native app, developers need to install the testing tools and frameworks of their choice, and follow these steps:
• Write test cases or scenarios that describe the expected behavior and outcome of the app or its components.
• Write test scripts or code that implement the test cases or scenarios using the testing tools and frameworks.
• Run the test scripts or code using the testing tools and frameworks, and observe the test results and reports.
• Fix any errors or bugs that are detected by the test scripts or code, and improve the app or its components accordingly.
• Repeat the process until the app or its components meet the desired quality, functionality, and performance standards.
For example:
// Import testing tools and frameworks
import React from 'react';
import { View, Text } from 'react-native';
import { render } from '@testing-library/react-native';
import renderer from 'react-test-renderer';
// Define a simple UI component
const Greeting = ({ name }) => {
return (
<View>
<Text>Hello {name}!</Text>
</View>
);
};
// Write a test case using React Native Testing Library
test('renders a greeting message', () => {
// Render the component with a name prop
const { getByText } = render(<Greeting name="Alice" />);
// Expect to find the text "Hello Alice!" in the component
expect(getByText('Hello Alice!')).toBeTruthy();
});
// Write a test case using Jest snapshot testing
test('matches snapshot', () => {
// Render the component with a name prop
const tree = renderer.create(<Greeting name="Alice" />).toJSON();
// Expect the component to match the snapshot
expect(tree).toMatchSnapshot();
});
15. How to optimize a React Native app?
Answer: Optimizing a React Native app is a process of improving the performance and efficiency of the app by reducing its size, memory usage, loading time, rendering time, etc. Optimizing a React Native app can be done at different stages, such as development stage, build stage, runtime stage, etc. There are different techniques and tools that can be used to optimize a React Native app, such as code splitting, tree shaking, minification, compression, caching, lazy loading, memoization, debouncing, throttling, etc. These techniques and tools have different effects and benefits, such as reducing bundle size, eliminating unused code, improving network requests, enhancing user experience, etc.
To optimize a React Native app, developers need to use the optimization techniques and tools of their choice, and follow these steps:
• Analyze the current performance and efficiency of the app or its components using performance analysis tools such as Chrome DevTools, React DevTools Profiler, Flipper, Hermes, etc.
• Implement the optimization techniques and tools using the documentation and guidelines provided by the framework or the tool.
• Measure the improvement and impact of the optimization techniques and tools using performance analysis tools and metrics such as bundle size, memory usage, loading time, rendering time, etc.
• Repeat the process until the app or its components reach the desired performance and efficiency standards.
16. What are the different ways to deploy a React Native app?
Answer: There are different ways to deploy a React Native app, depending on the target platform, the app requirements, and the developer preferences. Some of the common ways to deploy a React Native app are:
1. Using the native tools: This is the traditional way of deploying a React Native app, which involves using the native tools and platforms such as Xcode for iOS and Android Studio for Android. This way requires developers to have some knowledge and experience with native development, and to follow the specific steps and guidelines for each platform. This way also allows developers to access some native features and functionalities that are not available in other ways, such as push notifications, app icons, splash screens, etc.
2. Using Expo: Expo is a framework and a platform that simplifies the development and deployment of React Native apps. Expo provides a set of tools and services that enable developers to create and publish React Native apps without touching any native code or tools. Expo also provides some features and functionalities that are not available in the native tools, such as over-the-air updates, app sharing, app testing, etc. To use Expo, developers need to install expo-cli and expo-client packages, and follow these steps:
- Create a new React Native project using expo init <projectName> command.
- Develop the app using Expo APIs and components, and test it using Expo client app on a device or a simulator.
- Publish the app using expo publish command, which will upload the app bundle to Expo servers and generate a unique URL for the app.
- Build the app using expo build:ios or expo build:android command, which will create an IPA or APK file for the app using Expo servers.
- Distribute the app using App Store or Google Play Store, or using other methods such as TestFlight or HockeyApp.
3. Using CodePush: CodePush is a service that enables developers to deploy updates to React Native apps without going through the App Store or Google Play Store approval process. CodePush works by pushing the JavaScript code and assets of the app to a server, and then downloading them to the device when the app is launched. CodePush also allows developers to control when and how the updates are applied, such as mandatory or optional, immediate or on restart, etc. To use CodePush, developers need to install code-push-cli and react-native-code-push packages, and follow these steps:
- Create a new CodePush account and app using code-push register and code-push app add commands.
- Link the CodePush SDK to the React Native project using react-native link react-native-code-push command.
- Configure the CodePush options in the App.js file, such as deployment key, update mode, update dialog, etc.
- Release an update using code-push release-react <appName> <platform> command, which will bundle and upload the app code and assets to CodePush servers.
- Monitor and manage the updates using CodePush dashboard or commands.
17. What are the challenges of developing a React Native app?
Answer: Developing a React Native app can be challenging for various reasons, such as:
• Compatibility issues: React Native may not work on all devices or platforms, so developers should test their apps on a variety of devices and platforms to ensure compatibility. This is especially important for older devices or devices that use third-party libraries or services.
• Debugging challenges: Debugging React Native apps can be difficult, especially when the errors are related to the native code or the app’s performance. Developers may need to use a variety of tools and techniques to debug different parts of the app.
• Learning curve: React Native has a moderate learning curve, as it requires developers to have a good understanding of JavaScript, React, and native development. Developers may need to learn new concepts, tools, and best practices to use React Native effectively.
• Lack of native features: React Native does not support all the native features and functionalities that are available on iOS and Android platforms, such as advanced animations, gestures, camera, etc. Developers may need to use third-party libraries or write native modules to access these features.
• Maintenance issues: React Native is a fast-growing and evolving framework that may introduce breaking changes or deprecations in its updates. Developers may need to keep their apps up-to-date with the latest versions of React Native and its dependencies, which can be time-consuming and risky.
18. How to fix common React Native errors?
Answer: Here are some common React Native errors and how to fix them:
- Module not found: This error occurs when the module you are trying to import is not installed or the path to the module is incorrect. To fix this error, make sure the module is installed and the path is correct. You can use the `npm install` command to install a module.
- Syntax error: This error occurs when there is a syntax error in your code. To fix this error, check for syntax errors. You can use a linter to help you find syntax errors.
- ReferenceError: This error occurs when you try to use a variable or function that is not defined. To fix this error, make sure the variable or function is defined before use. You can use the `console.log()` function to see if the variable or function is defined.
- TypeError: This error occurs when you use a value incorrectly. To fix this error, make sure the value is used correctly. You can use the `console.log()` function to see the type of the value.
- NullReferenceError: This error occurs when you try to use a value that is null or undefined. To fix this error, make sure the value is not null or undefined before use. You can use the` isNan()` function to check if a value is null or undefined.
19. What are the best practices for developing React Native apps?
Answer: Some of the best practices for developing React Native apps are:
- Use functional components to write concise and testable code.
- Use TypeScript to avoid errors and make your code more readable.
- Use a linter to identify and fix errors in your code.
- Use a testing framework to test your app.
- Use a state management library to manage the state of your app.
- Use a code editor with React Native support to write and debug code more easily.
- Use a CI/CD pipeline to automate the build, test, and deployment of your app.
- Use a debugger to step through your code and inspect its values.
- Document your code to make it easier to understand and maintain.
- Follow the React Native style guide to write consistent and readable code.
20. What are the future trends in React Native?
Answer: React Native is an ever-evolving framework with several promising trends for the future:
- Web and Mobile Convergence: React Native is moving towards unifying web and mobile development. Tools like React Native Web enable code-sharing between web and mobile projects, reducing development effort and maintaining consistency across platforms.
- Improved Performance: Continuous efforts are being made to enhance the performance of React Native. This includes optimizing startup times, reducing memory consumption, and improving rendering speed. The introduction of Hermes, a JavaScript engine, has significantly boosted the runtime performance of React Native apps.
- Better Developer Experience: The React Native ecosystem is investing in improving the developer experience. Tools like Expo and Ignite CLI simplify project setup, provide debugging aids, and offer libraries that streamline common tasks.
- Cross-Platform Modules: As React Native matures, more cross-platform modules are being developed. These modules allow developers to write code that works seamlessly on both iOS and Android platforms, reducing the need for platform-specific code.
- Voice and AI Integration: With the rise of voice assistants and AI-powered applications, React Native is poised to facilitate seamless integration of voice recognition and AI features into mobile apps. Integrating with platforms like Amazon Alexa or Google Assistant becomes more accessible.
- GraphQL Adoption: GraphQL, a query language for APIs, is gaining popularity in the React Native community. Its efficiency in data fetching and flexibility in defining data requirements make it a valuable trend to watch.
21. How can you ensure good performance in a React Native app?
Answer: Ensuring good performance in a React Native app involves several strategies:
- Optimized Components: Use optimized and lightweight components to minimize rendering times. Memoization and PureComponent can help in this regard.
- Reduced Re-renders: Prevent unnecessary re-renders by utilizing shouldComponentUpdate, PureComponent, or React.memo for functional components.
- Async Rendering: Employ asynchronous rendering techniques such as React Native’s InteractionManager to schedule work during idle times, reducing frame drops.
- Hermes: Consider using Hermes, a JavaScript engine optimized for React Native, to improve runtime performance and reduce memory consumption.
- Profiler Tools: Utilize performance profiling tools like React Native Performance to identify bottlenecks and optimize specific parts of the app.
- Bundle Splitting: Implement bundle splitting to load only the required code for specific screens, improving initial load times.
- Image Optimization: Optimize images, use lazy loading, and consider using libraries like FastImage to handle images efficiently.
- State Management: Choose an efficient state management solution like Redux or MobX and avoid excessive state updates.
- Memory Management: Monitor and manage memory usage to prevent memory leaks and excessive garbage collection.
22. What is the significance of Hermes in React Native?
Answer: Hermes is a JavaScript engine optimized for running React Native apps. Its significance lies in its ability to enhance the performance of React Native applications in several ways:
- Improved Startup Time: Hermes significantly reduces the time it takes for a React Native app to start, resulting in a faster launch experience for users.
- Reduced Memory Consumption: It uses memory more efficiently, leading to lower memory consumption, reduced garbage collection, and fewer out-of-memory crashes.
- Smaller App Size: Hermes reduces the size of the JavaScript bundle, which can result in smaller app downloads and faster updates.
- Consistent JavaScript: It enforces stricter JavaScript standards, helping developers catch potential issues early in development.
- Compatibility: Hermes is compatible with most JavaScript libraries and frameworks commonly used in React Native development.
23. Can you explain the benefits of code-sharing between web and mobile in React Native?
Answer: Code-sharing between web and mobile in React Native offers several benefits:
- Reduced Development Time: Developers can reuse a significant portion of code, reducing the time required to build and maintain separate web and mobile applications.
- Consistent User Experience: Code-sharing ensures a consistent user interface and functionality across web and mobile platforms, enhancing the user experience.
- Unified Development Team: A single development team can work on both web and mobile applications, streamlining collaboration and knowledge sharing.
- Simplified Maintenance: Updates, bug fixes, and feature enhancements can be applied to both platforms simultaneously, reducing maintenance efforts.
- Cost-Efficiency: Code-sharing can lead to cost savings by eliminating the need for separate development teams and infrastructure.
- Cross-Platform Testing: Testing efforts are concentrated on a single codebase, simplifying quality assurance and reducing the need for platform-specific testing.
- Improved Scalability: As features and functionality grow, code-sharing enables efficient scaling across multiple platforms.
24. What are the different React Native frameworks?
Answer: React Native has various frameworks and tools that extend its capabilities and simplify development:
- Expo: Expo is a comprehensive framework and platform for React Native that provides a set of pre-configured tools, libraries, and services. It simplifies development by handling common tasks like building, testing, and deploying React Native apps.
- Ignite CLI: Ignite CLI is a command-line tool that helps developers quickly scaffold React Native projects with a predefined project structure. It offers boilerplates and generators for common app features, saving development time.
- React Native CLI: The official React Native Command Line Interface is a foundational tool for managing React Native projects. It enables project initialization, running and debugging apps, and generating platform-specific builds.
- MobX-State-Tree (MST): MST is a state management framework for React Native applications. It simplifies complex state management by providing a predictable and efficient state container.
25. When would you choose Expo over the React Native CLI for a project?
Answer: Expo and the React Native CLI each have their strengths, and the choice depends on the project’s requirements:
1. Choose Expo When:
- Rapid Prototyping: Expo is excellent for quickly prototyping apps, as it provides a simplified development environment with tools like Expo CLI, which handles many configuration aspects.
- Cross-Platform Apps: If your goal is to develop cross-platform apps for iOS and Android without extensive platform-specific code, Expo simplifies this process.
- Access to Managed Services: Expo offers access to managed services like push notifications and over-the-air updates, reducing the need for custom server setups.
- Developer Experience: Expo prioritizes developer experience, making it suitable for beginners and those who want to focus on coding without worrying about complex configurations.
2. Choose React Native CLI When:
- Custom Native Modules: If your project requires custom native modules or interactions with platform-specific APIs, React Native CLI provides more flexibility for native development.
- Advanced Configurations: When you need precise control over project configurations, dependencies, or specific build settings, React Native CLI offers a more open environment.
- Ejecting: React Native CLI allows “ejecting” from Expo if your project’s requirements change, giving you full control over the native code.
26. How does Ignite CLI improve the development process in React Native?
Answer: Ignite CLI enhances the React Native development process in the following ways:
- Boilerplate Templates: Ignite CLI provides boilerplate templates for common app structures, saving developers time in setting up projects and reducing repetitive tasks.
- Code Generation: It offers code generation for screens, components, and more. Developers can quickly scaffold new features with pre-configured templates.
- Best Practices: Ignite CLI follows best practices in React Native development, ensuring that projects start with a solid foundation in terms of code structure and organization.
- Plugin System: Ignite CLI is extensible through its plugin system. Developers can create or use existing plugins to add functionality or integrate with third-party services.
- Community Contributions: Ignite CLI benefits from contributions from the React Native community, incorporating improvements and features that streamline development.
27. What are the different React Native UI libraries?
Answer: React Native UI libraries offer a wide range of pre-designed components and styles to accelerate UI development. Here are some popular ones:
- NativeBase: NativeBase is a library that provides a set of essential UI components like buttons, cards, and navigation elements. It follows a consistent design language, making it easy to create cohesive user interfaces.
- React Native Elements: React Native Elements is a library that offers a variety of customizable UI components. It includes elements like buttons, icons, and form inputs, enabling rapid development with consistent styles.
- Shoutem UI: Shoutem UI is a highly customizable UI toolkit for React Native apps. It provides a rich set of components and allows developers to create unique and visually appealing interfaces.
- UI Kitten: UI Kitten is a UI framework that focuses on creating applications with a consistent design system. It includes modern components and styles that can be easily customized to match the desired look and feel.
28. How do UI libraries like NativeBase contribute to faster React Native development?
Answer: UI libraries like NativeBase can help speed up React Native development in a number of ways, following:
- Pre-Designed Components: NativeBase provides a set of pre-designed and highly customizable UI components such as buttons, cards, and navigation elements. Developers can use these components out of the box, saving time on UI implementation.
- Consistent Design Language: NativeBase follows a consistent design language, ensuring that the app’s UI maintains a cohesive and professional appearance across screens and platforms.
- Reduced Styling Effort: With NativeBase, developers often need to write less custom styling code, as the library’s components come with predefined styles that can be easily adjusted to match the app’s design.
- Modular and Extensible: NativeBase components are modular and can be easily extended or customized to meet specific design requirements, offering flexibility without sacrificing development speed.
- Community Contributions: Being a popular library, NativeBase benefits from community contributions, which means developers can leverage additional components and improvements created by the community.
By simplifying UI development and promoting code reuse, NativeBase accelerates the development of visually appealing and responsive React Native applications.
29. Can you explain the advantages of using React Native Elements in UI development?
Answer: React Native Elements offers several advantages when used in UI development for React Native applications:
- Wide Variety of Components: React Native Elements provides a comprehensive set of UI components, including buttons, icons, form inputs, and more. This extensive component library saves developers time and effort in building common UI elements.
- Customization: All components in React Native Elements are highly customizable. Developers can easily adjust the styles and appearance of components to match the app’s design requirements.
- Cross-Platform Compatibility: React Native Elements components are designed to work seamlessly on both iOS and Android platforms. This cross-platform compatibility ensures a consistent user experience.
- Responsive Design: The library includes responsive design principles, making it easier to create layouts that adapt to various screen sizes and orientations.
- Active Community: React Native Elements benefits from an active and supportive community, resulting in regular updates, bug fixes, and new features.
- Integration with Navigation Libraries: React Native Elements can be integrated with popular navigation libraries like React Navigation, enhancing the development of navigation-related UI components.
- Accessibility: The library emphasizes accessibility best practices, ensuring that components are usable by a wide range of users, including those with disabilities.
Overall, React Native Elements simplifies UI development in React Native projects by providing a versatile and customizable set of components that adhere to design standards and accessibility guidelines.
30. What are the different React Native debugging tools?
Answer: Debugging is a crucial part of the development process. React Native offers several tools to assist in debugging:
- React Native Debugger: React Native Debugger is a standalone debugger tool that provides a user-friendly interface for debugging React Native applications. It integrates with popular debugging tools like Redux DevTools and React DevTools.
- Flipper: Flipper is an extensible mobile app debugger developed by Facebook. It offers plugins that can be used for debugging React Native apps. Developers can inspect the app’s UI hierarchy, network requests, and logs.
- Redux DevTools: Redux DevTools is a browser extension that facilitates debugging Redux-based state management in React Native apps. It allows developers to trace actions, view state changes, and time-travel through the application’s state history.
31. What are the different React Native testing tools?
Answer: Testing is essential to ensure the reliability and functionality of React Native apps. Here are some testing tools used in React Native development:
- Jest: Jest is the default testing framework for React Native. It is designed for unit and component testing. Jest provides utilities for testing React components, including snapshot testing and mock functions.
- Detox: Detox is an end-to-end testing library for React Native applications. It allows for testing the entire app’s user interface and interactions across different devices and platforms.
- Enzyme: Enzyme is a JavaScript testing utility for React components. It provides a range of testing utilities to make it easier to assert, manipulate, and traverse React components’ output.
32. What are the different React Native deployment tools?
Answer: Deploying React Native apps involves building and distributing them to various platforms. Several tools assist in this process:
- Fastlane: Fastlane is an automation tool that streamlines the deployment pipeline for React Native apps. It automates tasks such as building, code signing, and distributing apps to app stores.
- App Center: App Center, provided by Microsoft, is a platform for building, testing, and distributing React Native apps. It offers a suite of services for continuous integration and delivery (CI/CD).
- Bitrise: Bitrise is a CI/CD platform that specializes in mobile app development. It provides workflows and integrations to automate the building, testing, and deployment of React Native apps.
33. What are the different React Native performance optimization tools?
Answer: Optimizing the performance of React Native apps is crucial for delivering a smooth user experience. Several tools can aid in this optimization:
- React Native Performance: React Native Performance is a library and toolset for monitoring and optimizing the performance of React Native apps. It provides insights into performance bottlenecks and offers recommendations for improvements.
- React Native Hermes: React Native Hermes is a JavaScript engine optimized for running React Native apps. It can significantly improve runtime performance and reduce memory usage.
- React Native Flipper: React Native Flipper is a tool that helps developers inspect and debug the performance of React Native apps. It offers plugins for profiling and analyzing app behavior.
34. Describe the purpose of ‘props’ in React Native
Ans: In React Native, ‘props’ or properties serve as a means of passing data from one component to another. They play a crucial role in creating dynamic and reusable components. When a parent component wants to share information with its child components, it does so through ‘props’. This facilitates the development of flexible and modular user interfaces.
35. How do you handle user input using React Native?
Ans:Handling user input in React Native involves utilizing various UI components and event handlers. For instance, when working with text input, we use the TextInput
component, and we respond to user interactions through event handlers like onChangeText
. This ensures that the app can capture and respond to user input effectively, providing a seamless user experience.
36. Explain the concept of ‘flexbox’ and its role in React Native layout.
Ans: ‘Flexbox’ in React Native is a layout model that simplifies the arrangement of items within a container. It allows for the creation of responsive and flexible layouts. Think of it as a set of rules for distributing space among components. Properties like flexDirection
, justifyContent
, and alignItems
help control the placement and alignment of components, making it easier to create visually appealing and adaptable designs.
37. Describe the purpose of ‘AsyncStorage’ in React Native.
Ans: ‘AsyncStorage’ serves as a simple, asynchronous, persistent storage system in React Native. Its primary purpose is to store small amounts of data locally on the device. This can include information like user preferences, authentication tokens, or cached data. The ‘Async’ aspect means that these storage operations don’t block the main thread, ensuring smooth app performance.
38. How do you handle form validation in React Native?
Ans: Form validation in React Native involves ensuring that user input meets specific criteria before submission. Typically, this is achieved by defining rules for validation and checking user input against these rules. Events like onBlur
or onChangeText
are used to trigger validation logic. Error messages are then displayed to guide users when their input doesn’t meet the required criteria.
39. How can you handle offline storage in a React Native app?
Ans: Offline storage in React Native involves persisting data locally on the device to ensure accessibility even when the app is offline. Developers commonly use solutions like ‘AsyncStorage’ for smaller datasets or implement more robust solutions such as SQLite or Realm for larger and more complex data structures. This allows the app to maintain functionality and provide a seamless experience even without an internet connection.
40. What is the purpose of the ‘PixelRatio’ module in React Native?
Ans: The ‘PixelRatio’ module in React Native is designed to handle different screen densities and resolutions. Its purpose is to assist in creating responsive designs that look consistent across devices with varying pixel densities. By using ‘PixelRatio’ methods, such as getPixelSizeForLayoutSize
and getFontScale
, developers can adjust sizes and layouts to ensure a visually consistent experience across devices with different pixel densities. This helps maintain a uniform look and feel for the app on various screens.
Conclusion,
In this blog post, we have learned about some of the most important interview questions on React Native that can help you assess your understanding and depth on the topic. We have covered most of the common asked React Native interview questions and answers.
We hope you have enjoyed this blog post and found it useful and informative. If you want to learn more about React Native or other topics related to web development and mobile development , you can check our website or visit our Academy. If you have any feedback or questions about this blog post, feel free to leave a comment below. We would love to hear from you.