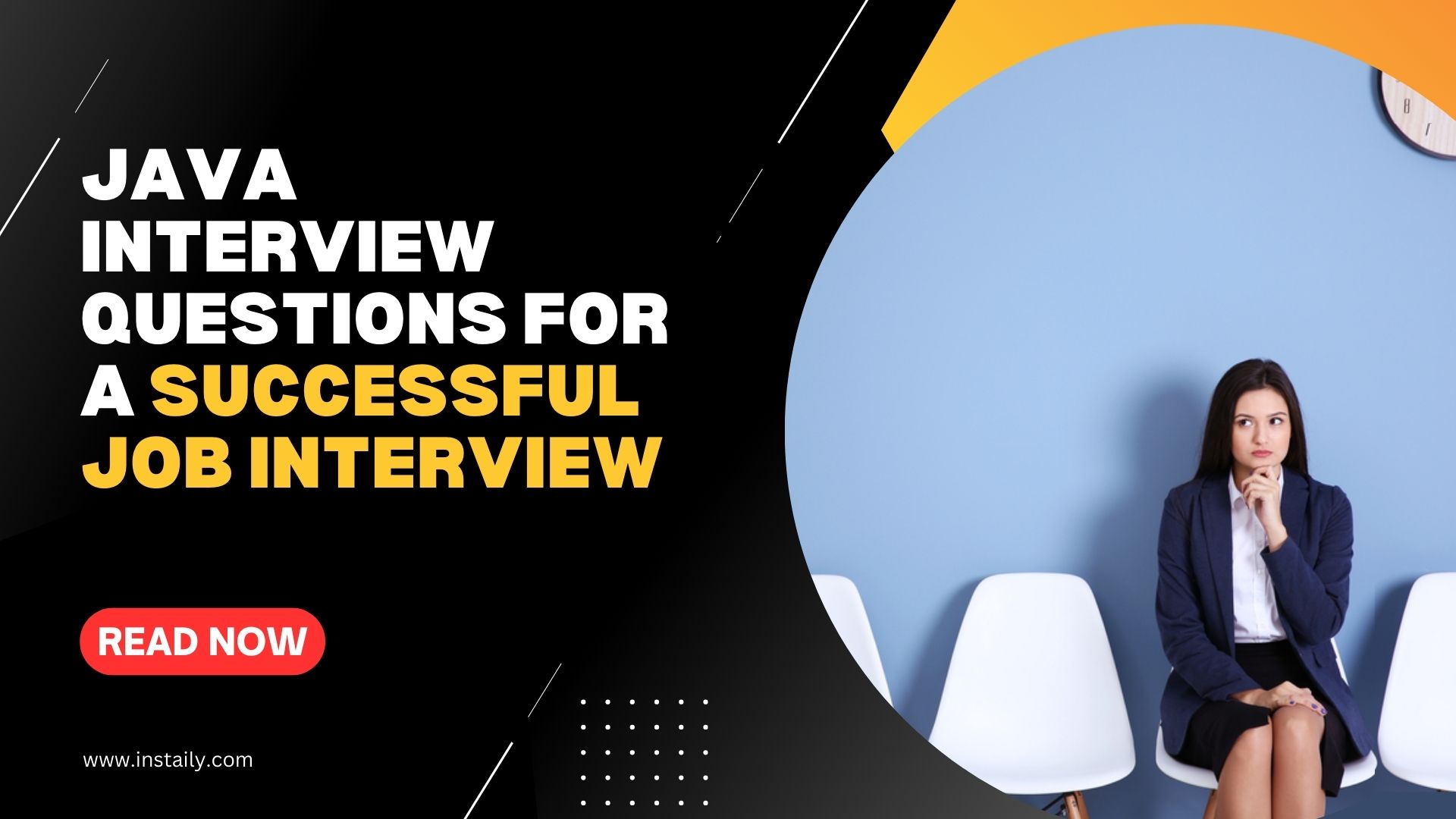
Java Interview Questions and Answers
Embark on your journey to becoming a Java master and ace your next interview with Instaily Academy’s comprehensive compilation of Java interview questions and answers. This meticulously crafted guide, tailored for both beginners and experienced professionals, will equip you with the knowledge and confidence to navigate any Java interview scenario with ease.
Master Java Interview Questions for Freshers and Experts
Whether you’re a seasoned Java professional or just starting out, this resource delves into the most commonly asked Java interview questions, providing insightful and detailed explanations for each. Stay ahead of the curve with our regularly updated collection of new Java interview questions and answers, ensuring you’re well-prepared for the ever-evolving industry demands.
Sharpen your Java skills, gain a deeper understanding of the language, and impress your potential employers with Instaily Academy’s expert-led guidance. This comprehensive resource is your key to unlocking a successful career in the dynamic world of Java programming.
Q1. What makes Java a platform-independent programming language?
Ans: Java’s platform independence is rooted in its compilation to bytecode. The Java compiler translates source code into an intermediate form, bytecode, which is not tied to any specific hardware or operating system. This bytecode is then executed on any device equipped with a Java Virtual Machine (JVM). The JVM acts as an abstraction layer, interpreting the bytecode and facilitating seamless execution across diverse platforms. This eliminates the need for recompilation, enabling the “write once, run anywhere” paradigm and making Java a versatile and platform-independent programming language.
Q2. What are the data types supported by Java?
Ans: There are 8 primitive data types that are supported by Java:
- byte
- int
- short
- long
- float
- double
- char
- boolean
Q3. Highlight the key features of Java.
Ans: Java’s standout features encompass platform independence, realized through bytecode execution on a Java Virtual Machine (JVM). Its object-oriented paradigm promotes modular and reusable code. The language stands out for its simplicity, featuring a clean syntax that aids developer productivity. Automatic memory management, facilitated by garbage collection, relieves developers from manual memory handling concerns. Furthermore, Java excels in concurrent programming with built-in multithreading support, enhancing application performance. These robust features collectively contribute to Java’s widespread adoption and enduring relevance in the software development landscape.
Q4. Explain autoboxing and unboxing in Java.
Ans: Autoboxing in Java refers to the automatic conversion of primitive data types to their corresponding wrapper classes. For instance, converting an int to an Integer. On the other hand, unboxing involves the automatic conversion of wrapper class objects back to their primitive data types. Java performs these conversions seamlessly, simplifying code and enhancing flexibility when working with both primitive types and their object representations. Autoboxing and unboxing contribute to the language’s ease of use and promote a more unified approach to handling data.
Q5. Differentiate between overloading and overriding in Java.
Ans: In Java, overloading occurs when a class has multiple methods with the same name but different parameter types or a different number of parameters. Overloading allows methods to be distinguished based on their signatures.
On the other hand, overriding happens in a subclass that provides a specific implementation for a method that is already defined in its superclass. The overriding method in the subclass must have the same signature (method name, return type, and parameters) as the method in the superclass.
Q6. How do HashSet and TreeSet classes differ in Java?
Ans: HashSet and TreeSet are both implementations of the Set interface, but they differ in their underlying data structures and ordering:
HashSet:
- Uses a hash table for storage.
- Provides constant-time complexity for basic operations (add, remove, contains).
- Unordered collection, making it faster for general use.
- No guarantee of elements being stored in any specific order.
TreeSet:
- Implemented as a Red-Black tree.
- Offers log(n) time complexity for basic operations.
- Maintains elements in sorted order (natural order or specified comparator).
- Additional navigational methods for tree-based operations.
Q7. Define a list and explain the distinction between ArrayList and LinkedList.
Ans: A list is an ordered collection of elements.
ArrayList:Vector
- Implemented as a dynamic array, allowing fast random access.
- Efficient for retrieving elements by index.
- Slower for insertions and deletions, as it requires shifting elements.
LinkedList:
- Implemented as a doubly-linked list, excelling in insertions and deletions.
- Efficient for sequential access but slower for random access.
- Node-based structure, providing faster insertions and deletions in the middle of the list.
Choose between ArrayList and LinkedList based on the specific requirements of your use case.
Q8. Provide definitions for JVM, JDK, and JRE.
Ans: JVM (Java Virtual Machine): It’s a virtual machine that executes Java bytecode, providing a platform-independent runtime environment for Java applications.
JDK (Java Development Kit): It is a software development kit containing tools for developing, debugging, and monitoring Java applications, including the JRE.
JRE (Java Runtime Environment): It encompasses the JVM and libraries, providing the necessary runtime support for executing Java applications but lacking development tools found in the JDK.
Q9. Elaborate on Java’s WORA (Write Once, Run Anywhere) concept.
Ans: Java’s WORA (Write Once, Run Anywhere) concept signifies that once a Java program is written and compiled into bytecode, it can run on any device with a compatible Java Virtual Machine (JVM). The bytecode serves as an intermediary representation that is independent of the underlying hardware or operating system. This platform independence allows developers to create Java applications without concern for the specific environment where the code will be executed. The JVM acts as a universal interpreter, ensuring that the same Java program can be executed seamlessly across diverse platforms, promoting code reusability and reducing the need for platform-specific adaptations.
Q10. Differentiate between StringBuffer and StringBuilder in Java.
Ans: Both StringBuffer and StringBuilder are used for string manipulation, but they differ in terms of synchronization and performance:
StringBuffer:
- Thread-safe due to synchronized methods.
- Slower performance in a multi-threaded environment.
- Ideal for scenarios requiring thread safety, such as in a multi-threaded application.
StringBuilder:
- Not thread-safe, as methods are not synchronized.
- Better performance in a single-threaded environment.
- Suitable when thread safety is not a concern, offering faster execution for individual threads.
Q11. Explain the scenarios when you override hashcode() and equals() methods.
Ans: Override hashCode()
and equals()
methods in Java when:
HashMap or HashSet Usage:
- Overriding
hashCode()
ensures proper functioning of hash-based collections like HashMap and HashSet. - Overriding
equals()
ensures correct comparison of objects within these collections.
Custom Object Equality:
- When comparing objects for equality based on their content, not their memory reference.
- This is crucial for accurate usage of collections that rely on equality, like removing duplicates in a Set.
Q12. Discuss the potential issues if hashcode() is not overridden.
Ans: If hashCode()
is not overridden when necessary, it can lead to several issues:
Incorrect HashMap/HashSet Behavior:
- Inconsistent or incorrect behavior in collections like HashMap and HashSet.
- Failure to locate objects in hash-based data structures, resulting in unexpected behavior.
Unequal Objects May Have Different Hash Codes:
- Objects with the same content may produce different hash codes, violating the contract between
hashCode()
andequals()
. - This can lead to incorrect equality comparisons.
Performance Impact:
- Reduced performance in hash-based collections due to inefficient hashing.
- Increased likelihood of hash collisions, leading to degraded performance.
To avoid these issues, it’s crucial to override hashCode()
whenever equals()
is overridden, ensuring consistent behavior in hash-based collections.
Q13. Define immutable classes in Java.
Ans: Immutable classes in Java are classes whose instances cannot be modified after creation. Once an immutable object is instantiated, its state, represented by its fields, cannot be changed. Instead of modifying the object, any operation on an immutable class creates and returns a new object with the desired state. Immutability ensures thread safety, simplifies reasoning about code, and facilitates the creation of predictable and reliable software. Examples of immutable classes in Java include String and the wrapper classes for primitive types like Integer and Double.
Q14. List the access specifiers used in Java.
Ans: There are four access specifiers:
Public:
- Accessible from anywhere. No restrictions on visibility.
Private:
- Accessible only within the same class. Restrictions on visibility outside the class.
Protected:
- Accessible within the same package and by subclasses, even if they are in different packages.
Default (Package-Private):
- Accessible only within the same package. No access from outside packages without inheritance.
These access specifiers control the visibility and accessibility of classes, methods, and fields in Java.
Q15. Explain the concept of singleton in Java.
Ans: A singleton is a design pattern that ensures a class has only one instance and provides a global point of access to it. The singleton pattern involves defining a class with a method that creates an instance of the class if one does not exist, and if an instance already exists, it returns the reference to that instance. This guarantees that only one instance of the class is created and shared across the entire application. Singletons are often used for logging, driver objects, caching, thread pools, or database connections, where having multiple instances would be detrimental.
Q16. Are all try blocks in Java mandatory to have corresponding catch blocks?
Ans: No, all try blocks in Java are not mandatory to have corresponding catch blocks. A try block can be followed by either a catch block, a finally block, or both. The catch block is used to handle exceptions that occur within the try block, while the finally block contains code that is guaranteed to execute, whether an exception is thrown or not. It is valid to have a try block without a catch block if the intention is to only execute the code in the finally block for cleanup or resource release purposes.
Q17. Explain the role of the final keyword in Java.
Ans: The final
keyword serves various purposes in Java:
Final Variable:
- When applied to a variable, it makes the variable’s value unmodifiable, acting as a constant.
Final Method:
- When applied to a method, it prevents the method from being overridden by subclasses.
Final Class:
- When applied to a class, it prevents the class from being extended, making it non-inheritable.
Using final
ensures immutability, restricts method modification, and prevents class extension, contributing to code safety and design integrity.
Q18. Can a class be declared abstract without an abstract method?
Ans: Yes, a class can be declared abstract in Java without containing any abstract methods. An abstract class can have concrete (non-abstract) methods along with abstract methods. Abstract classes provide a way to define common functionality and structure for their subclasses. While it is common for abstract classes to have abstract methods, it is not a strict requirement. The presence of abstract methods in a class determines whether the class itself needs to be declared as abstract.
Q19. Differentiate between an abstract class and an interface in Java, citing five key distinctions.
Ans: 1. Constructors:
- Abstract Class: Can have constructors, allowing initialization of instance variables.
- Interface: Cannot have constructors since interfaces represent a contract rather than an instantiated object.
2. Methods:
- Abstract Class: Can have both abstract and concrete methods.
- Interface: Contains only abstract methods until Java 8, but can have default and static methods from Java 8 onwards.
3. Multiple Inheritance:
- Abstract Class: Supports single inheritance as a class can extend only one abstract class.
- Interface: Supports multiple inheritance as a class can implement multiple interfaces.
4. Fields:
- Abstract Class: Can have instance variables (fields).
- Interface: Fields are implicitly public, static, and final (constants).
5. Access Modifiers:
- Abstract Class: Can have various access modifiers for methods (public, protected, private).
- Interface: All methods are implicitly public and abstract; members are public, static, and final.
Choosing between an abstract class and an interface depends on the specific design requirements and the need for multiple inheritance.
Q20. Define serialization and explain when it should be used.
Ans: Serialization in Java is the process of converting an object into a byte stream, allowing it to be easily stored, transmitted, or reconstructed. This byte stream can be written to a file, sent over a network, or stored in a database.
Use Serialization When:
1. Object Persistence:
- Saving the state of an object for later retrieval, especially in scenarios like storing the state of an application between sessions.
2. Network Communication:
- Transmitting objects between applications over a network.
3. Database Storage:
- Storing object data in databases, especially in scenarios where complex data structures need to be saved.
4. Cross-Language Communication:
- Facilitating communication between Java and non-Java systems by converting objects into a standard format for data interchange.
Serialization is essential when the need arises to convert complex object structures into a format suitable for storage or transmission.
Q21. Elaborate on multithreading and its implementation methods in Java.
Ans: Multithreading in Java:
Multithreading is the concurrent execution of two or more threads, allowing for parallel processing and improved program efficiency.
Implementation Methods:
1. Extending the Thread class:
- Create a new class that extends the
Thread
class. - Override the
run()
method to define the code to be executed by the thread. - Instantiate an object of the new class and call its
start()
method to begin execution.
class MyThread extends Thread {
public void run() {
// Code to be executed concurrently
}
}
2. Implementing the Runnable interface:
- Create a class implementing the
Runnable
interface. - Implement the
run()
method with the code to be executed. - Create a
Thread
object, passing an instance of the class implementingRunnable
to its constructor.
class MyRunnable implements Runnable {
public void run() {
// Code to be executed concurrently
}
}
// Creating a thread
Thread myThread = new Thread(new MyRunnable());
Both methods enable the execution of code concurrently, providing flexibility and control over multithreading in Java. The choice between them depends on whether the class needs to extend another class (use Thread) or implement multiple interfaces (use Runnable).
Q22. Define collection and the collections framework in Java.
Ans: Collection in Java:
A collection in Java is an object that groups multiple elements into a single unit. Collections provide a way to organize, store, and manipulate groups of objects.
Collections Framework:
The Collections Framework in Java is a set of classes and interfaces in the java.util
package that provide high-performance, reusable data structures. It includes interfaces like List
, Set
, and Map
, along with their implementing classes such as ArrayList
, HashSet
, and HashMap
. The framework supports the manipulation of collections and offers a standard way to work with data structures, making it easier to design and implement algorithms that involve collections of objects.
Q23. Explain the BlockingQueue concept in the Java collections framework.
Ans: A BlockingQueue
in Java is a queue that supports operations that wait for the queue to become non-empty when retrieving elements or non-full when storing elements. It is part of the Java Collections Framework and is particularly useful in concurrent programming scenarios.
Key Methods:
1. put(E element):
- Adds the specified element to the queue.
- If the queue is full, it waits until space becomes available.
2. take():
- Retrieves and removes an element from the head of the queue.
- If the queue is empty, it waits until an element becomes available.
BlockingQueue implementations help in scenarios where producers and consumers need to synchronize their operations, ensuring smooth communication and coordination between threads in a multithreaded environment.
Q24. Differentiate between ArrayList and Vector in Java.
Ans:
Feature | ArrayList | Vector |
Synchronization | Not synchronized | Synchronized |
Performance | Generally faster | Slower due to synchronization |
Capacity Increment | Increases by 50% | Doubles its size |
Thread Safety | Not inherently thread-safe | Inherently thread-safe, but with performance impact |
Legacy Status | Introduced in Java 1.2 | Part of original Java (JDK 1.0), somewhat legacy |
In most modern applications, ArrayList is preferred due to its better performance unless explicit thread safety is required, in which case Vector may be considered.
Q25. Define constructors and constructor overloading in Java.
Ans: Constructors in Java:
Constructors in Java are special methods that initialize objects when they are created. They have the same name as the class and do not have a return type. Constructors are invoked using the new
keyword when an object is instantiated.
Example:
public class MyClass {
// Default Constructor
public MyClass() {
// Initialization code
}
// Parameterized Constructor
public MyClass(int parameter) {
// Initialization code with parameter
}
}
Constructor Overloading:
Constructor overloading in Java refers to the practice of defining multiple constructors in a class, each with a different parameter list. This allows objects to be initialized in various ways based on the provided arguments.
Example:
public class Person {
String name;
int age;
// Default Constructor
public Person() {
// Initialization code
}
// Parameterized Constructor
public Person(String name) {
this.name = name;
}
// Another Parameterized Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Constructor overloading enhances the flexibility of object creation by providing multiple ways to initialize an object.
Q26. Differentiate between enumeration and iterator in Java.
Ans:
Feature | Enumeration | Iterator |
Introduced In | Legacy (Enumeration introduced in JDK 1.0) | Modern (Iterator introduced in JDK 1.2) |
Methods | Limited methods (hasMoreElements() , nextElement() ) | More methods (hasNext() , next() , remove() in the Iterator interface) |
Fail-Safe/Fail-Fast | Fail-Safe (Enumeration doesn’t throw ConcurrentModificationException ) | Fail-Fast (Iterator throws ConcurrentModificationException if the collection is modified during iteration) |
Q27. Explain the purpose of the break keyword in switch statements.
Ans: The break
keyword in switch statements is used to terminate the switch block. When a break
statement is encountered, it exits the switch block, preventing fall-through to subsequent cases. It helps control the flow of execution and avoids unnecessary evaluation of subsequent cases.
Example:
switch (day) {
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday");
break;
// ...
default:
System.out.println("Invalid day");
}
Q28. List the different states of a thread in Java.
Ans: Thread states in Java include:
- NEW: A thread that has been created but not yet started.
- RUNNABLE: A thread executing in the Java Virtual Machine.
- BLOCKED: A thread waiting to acquire a lock.
- WAITING: A thread waiting indefinitely for another thread to perform a particular action.
- TIMED_WAITING: A thread waiting for another thread to perform a particular action for a specified waiting time.
- TERMINATED: A thread that has exited.
Q29. Is it true that Java programs are immune to memory leaks due to garbage collection?
Ans: No, Java programs are not immune to memory leaks. While garbage collection helps manage memory by reclaiming unused objects, memory leaks can still occur if references to objects are unintentionally kept, preventing them from being garbage collected. It’s essential for developers to manage resources carefully, especially in long-running applications, to prevent memory leaks.
Q30. Explain object cloning in Java.
Ans: Object cloning in Java involves creating an exact copy of an object. The Cloneable interface is used to indicate that an object can be cloned, and the clone()
method is used to perform the cloning. It creates a new object with the same attributes as the original.
Example:
public class MyClass implements Cloneable {
// ...
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
Keep in mind that the default clone()
method performs a shallow copy. For a deep copy, custom implementation is required.
Q31. Are the throw and throws keywords interchangeable? If not, explain the difference.
Ans: No, the throw
and throws
keywords are not interchangeable.
- throw: Used to explicitly throw an exception in a program.
throw new SomeException("This is an exception");
- throws: Used in method signatures to declare that the method may throw certain exceptions. It doesn’t throw an exception itself but indicates which exceptions might be thrown.
void myMethod() throws SomeException {
// method code
}
Q32. Differentiate between JDK, JRE, and JVM.
Ans:
Component | Description |
JDK (Java Development Kit) | Includes the tools and binaries required for Java development, such as the compiler (javac ), debugger, and libraries. |
JRE (Java Runtime Environment) | Provides the runtime environment needed to run Java applications. It includes the JVM and essential libraries but lacks development tools. |
JVM (Java Virtual Machine) | Executes Java bytecode, providing platform independence. It interprets or compiles bytecode into machine-specific instructions during runtime. |
Q33. Differentiate between an inner class and a subclass in Java.
Ans:
Feature | Inner Class | Subclass |
Enclosing Context | Exists within another class or method. | Derived from a parent class using extends . |
Access to Members | Has access to the members of the enclosing class, including private members. | Inherits members from the parent class, excluding private members. |
Object Creation | Requires an instance of the outer class for instantiation. | Can be instantiated independently. |
Static Context | Can be static or non-static. | Can be static or non-static. |
Relationship | Often used for logical grouping or encapsulation of functionality within a class. | Represents an “is-a” relationship, where a subclass is a specialized version of its superclass. |
Q34. Identify the base class from which all classes in Java are derived.
Ans: The base class from which all classes in Java are derived is java.lang.Object
. Every class in Java is a direct or indirect subclass of this class. The Object
class provides fundamental methods like toString()
, equals()
, and hashCode()
, which can be overridden in user-defined classes.
Q35. Differentiate between an abstract class and an interface in Java.
Ans:
Feature | Abstract Class | Interface |
Constructors | Can have constructors, including parameterized ones. | Cannot have constructors. |
Method Implementation | Can have both abstract and concrete methods. | All methods are implicitly abstract. |
Fields | Can have instance variables (fields). | Fields are implicitly public, static, and final (constants). |
Access Modifiers | Supports various access modifiers for methods. | All methods are implicitly public. |
Multiple Inheritance | Supports single inheritance. | Supports multiple inheritance through interfaces. |
Keyword | Use abstract keyword. | Use interface keyword. |
Q36. Define a platform.
Ans: A platform, in the context of Java, refers to the combination of hardware and software that provides an environment for executing Java programs. It consists of two main components:
- Hardware Platform: The physical computing machinery where Java programs run (e.g., a computer, smartphone, or embedded device).
- Software Platform: The Java Virtual Machine (JVM) and the associated runtime libraries. The JVM interprets and executes Java bytecode, making Java applications platform-independent.
Q37. Explain two methods for executing code before the main method in Java.
Ans: Two common methods for executing code before the main
method are:
1. Static Block:
class MyClass {
static {
// Code to be executed before main
}
public static void main(String[] args) {
// Main method code
}
}
2. Initialization Block:
class MyClass {
{
// Code to be executed before main
}
public static void main(String[] args) {
// Main method code
}
}
Both static and initialization blocks are executed when the class is loaded, allowing code to run before the main
method.
Q38. Discuss the benefits of using inheritance in Java.
Ans:
- Code Reusability: Inheritance allows the reuse of code from existing classes, promoting a more modular and maintainable codebase.
- Polymorphism: Inheritance enables polymorphic behavior, where objects of derived classes can be treated as objects of their base class, enhancing flexibility.
- Method Overriding: Subclasses can override methods of the superclass, allowing customization of behavior without modifying the original code.
- Structural Hierarchy: Inheritance creates a clear structural hierarchy, making the relationships between classes more evident and improving code organization.
Q39. Differentiate between a stack and a queue in Java.
Ans:
Feature | Stack | Queue |
Order of Elements | Last In, First Out (LIFO) | First In, First Out (FIFO) |
Insertion Operation | Push (adds to the top of the stack) | Enqueue (adds to the rear of the queue) |
Removal Operation | Pop (removes from the top of the stack) | Dequeue (removes from the front of the queue) |
Example in Java | Stack<E> class | LinkedList<E> class with addFirst() and removeFirst() methods |
Q40. Identify types of exceptions caught at compile time.
Ans: Exceptions caught at compile time in Java are checked exceptions. Examples include:
- IOException
- SQLException
- ClassNotFoundException
These exceptions are checked by the compiler, and the code must either handle them using a try-catch
block or declare that the method throws the exception using the throws
clause.
Q41. Is String a data type in Java?
Ans: In Java, String
is not a primitive data type; it is a class. However, it is widely used and treated as a fundamental data type due to its special handling in the language.
Q42. List five key features of Java.
Ans:
- Platform Independence (WORA): Write Once, Run Anywhere.
- Object-Oriented: Encourages the use of classes and objects.
- Robust and Secure: Memory management, exception handling, and access controls enhance reliability.
- Multi-threading: Supports concurrent execution of multiple threads.
- Rich Standard Library: Comprehensive set of libraries and APIs for various tasks.
Q43. Explain why Strings in Java are considered immutable.
Ans: Strings in Java are considered immutable because once a String
object is created, its state (the sequence of characters) cannot be changed. Any operation that appears to modify a String
actually creates a new String
object. This immutability has several advantages, including thread safety, security, and improved performance due to caching.
Q44. Differentiate between an array and Vector in Java.
Ans:
Feature | Array | Vector |
Size Flexibility | Fixed size once declared. | Resizable; can dynamically grow or shrink. |
Synchronization | Not synchronized (no thread safety). | Synchronized (thread-safe operations). |
Performance | Generally faster. | Slower due to synchronization overhead. |
Type Safety | Supports primitive and object types. | Supports only object types. |
Implementation Class | Part of the core Java language. | Part of the Java Collections Framework. |
Q45. Explain why Java is considered a dynamic language.
Java is often considered a dynamic language due to its support for dynamic features, including:
- Dynamic Memory Allocation: Objects are allocated memory dynamically at runtime.
- Reflection: Allows inspection and modification of program elements (classes, methods, fields) at runtime.
- Dynamic Loading: Classes can be loaded and instantiated dynamically during program execution.
While Java is statically typed (types are checked at compile time), its dynamic features provide flexibility and adaptability during runtime, making it more dynamic than languages that lack such features.